How to Run Python Scripts: A Beginner's Guide
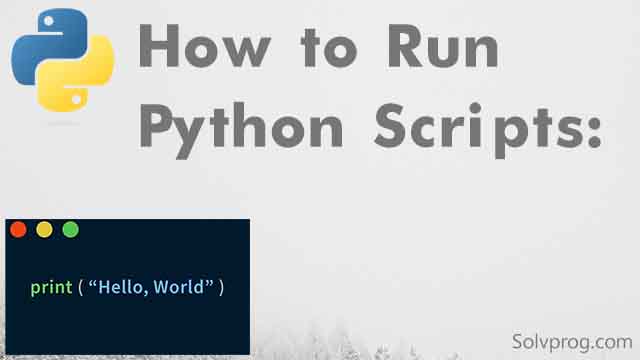
Learn the best ways to run Python code and get started with your own scripts. Step-by-Step Instructions for Running Python Scripts on Windows, Mac, and Linux.
Here are some step-by-step instructions that will help you run Python scripts on Windows, Mac, and Linux:
- Install Python on your computer: You can download the latest version of Python from the official website at https://www.python.org/downloads/. Make sure to choose the appropriate version for your operating system.
- Choose a text editor or IDE: A text editor or IDE will allow you to create, edit, and run your Python scripts. Popular options include Visual Studio Code, PyCharm, Sublime Text, Atom, and Notepad++. Choose one that you find comfortable to use.
- Write your Python code: Create a new file in your text editor and write your Python code. Be sure to save the file with a .py. extension.
- Open a terminal or command prompt: On Windows, press the Windows key + R and type cmd, then press Enter. On Mac, open the Terminal app. On Linux, press Ctrl + Alt + T.
- Navigate to the directory where your Python file is located: Use the cd command to navigate to the directory where your Python file is located. For example, if your file is located on the desktop, type cd Desktop.
- Run your Python script: Type python followed by the name of your Python file and press Enter. For example, if your file is named myscript.py, type python myscript.py and press Enter.
- View the output: If your script produces any output, it will be displayed in the terminal.
By following these steps, you should be able to run Python scripts on Windows, Mac, and Linux.
Simple example code to run Python code for the first time:
Python: hello world program syntax
print("Hello, World!")
- Open a text editor such as Notepad or Sublime Text and paste the preceding code into it.
- Save the file with a .py extension, such as hello.py.
- Go to the location where you stored the file using a command prompt or terminal.
- Enter python hello.py into the command line.
- The output Hello, World! should be displayed on the terminal.
This is simply a basic example of how to run Python code. To learn more interesting activities, you can edit the code or develop your own scripts. As I am just going begin doing some great things ahead.
Here are some more examples to actually see how you can run Python code to solve and get the desired results:
1. Calculate the sum of two numbers:
a = 5
b = 10
sum = a + b
print("The sum of", a, "and", b, "is", sum)
Solution:
The sum of 5 and 10 is 15
2. Create a list of numbers and print out the even ones:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = []
for num in numbers:
if num % 2 == 0:
even_numbers.append(num)
print("Even numbers:", even_numbers)
Solution:
Even numbers: [2, 4, 6, 8, 10]
3. Convert Celsius to Fahrenheit:
celsius = 25
fahrenheit = (celsius * 9/5) + 32
print(celsius, "degrees Celsius is equal to", fahrenheit, "degrees Fahrenheit")
Solution:
25 degrees Celsius is equal to 77.0 degrees Fahrenheit
4. Create a dictionary of fruits and their prices, and print out the most expensive fruit:
fruits = {"apple": 0.50, "banana": 0.25, "orange": 0.75, "grape": 1.00}
most_expensive_fruit = max(fruits, key=fruits.get)
print("The most expensive fruit is", most_expensive_fruit, "which costs $", fruits[most_expensive_fruit])
Solution:
The most expensive fruit is grape which costs $ 1.0
These examples cover a range of basic programming concepts in Python, including variables, data types, loops, conditionals, functions, and dictionaries. Give them a try and see what you can do with Python!
A Step-by-step short guide to run a Python script:
1. Open a text editor such as Sublime Text, Visual Studio Code, PyCharm, or IDLE.
2. Write your Python code in the editor and save it with a .py extension. For example, you can create a file called "hello.py" and write the following code:
print("Hello, World!")
3. Open a command prompt or terminal on your computer.
4. Navigate to the directory where the Python script is saved. You can use the cd command to change directories. For example, if your Python script is saved in the Documents folder, you can navigate to that directory by typing:
cd Documents
5. Once you are in the correct directory, type the following command to run the Python script:
python hello.py
6. Press Enter and the Python interpreter will execute the code in the script.
7. You should see the output "Hello, World!" displayed in the command prompt or terminal.
In summary, Python is a popular and easy-to-learn programming language that can be used for a variety of tasks, from simple scripts to complex applications. To get started with running Python code, you need to install Python on your computer and choose a text editor or IDE. Then, you can write your Python code, save it with a .py extension, and run it using the python command in a terminal or command prompt. Some simple examples of Python code that you can try include calculating the sum of two numbers, creating a list of numbers and printing out the even ones, converting Celsius to Fahrenheit, and creating a dictionary of fruits and their prices. These examples cover a range of basic programming concepts in Python, and you can modify them or write your own scripts to perform more complex tasks.