Display Output in JavaScript - JavaScript Tutorial
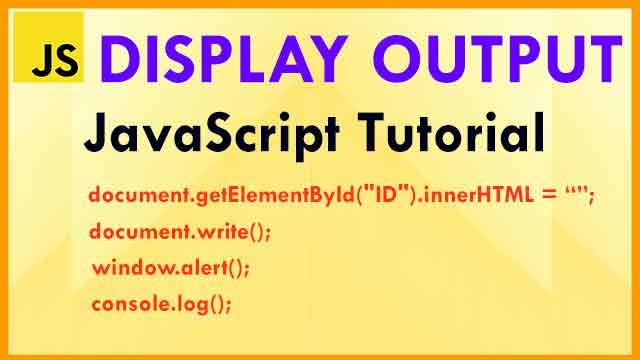
We can use JavaScript to "display" data in various ways. But, each statement has a specific purpose to use for displaying an output.
We will be using four statements types to display output in JavaScript.
- 1. Using innerHTML.
- 2. Using document.write().
- 3. Using window.alert().
- 4. Using console.log().
Using innerHTML
An HTML element is selected by id selector attribute using the document.getElementById(id) method.
The id attribute defines the HTML element. The innerHTML property defines the HTML content.
<!DOCTYPE html>
<html>
<body>
<h1>Display Output - JavaScript Tutorial</h1>
<p id="first-id"></p>
<script>
document.getElementById("first-id").innerHTML = "Sagar InspireMe";
</script>
</body>
</html>
Using document.write()
For testing purposes only, we use document.write():
<!DOCTYPE html>
<html>
<body>
<h1>Display Output - JavaScript Tutorial</h1>
<script>
document.write("Used for testing purpose only..");
</script>
</body>
</html>
Using document.write() deletes all existing HTML when loaded on the page.
<!DOCTYPE html>
<html>
<body>
<h1>Click the button, all existing HTML erases</h1>
<button type="button" onclick="document.write('deleted..')">Try this button</button>
</body>
</html>
Using window.alert()
<html>
<body>
<h1>Display Output - JavaScript Tutorial</h1>
<script>
window.alert("window.alert method is working..");
alert("alert method is working..");
alert("window.alert & alert are the same.. you can use any of it!");
</script>
</body>
</html>
Using console.log()
console.log() method is used for debugging purposes, you can inspect element and view the output in developer tools settings (console) in your browser.
<!DOCTYPE html>
<html>
<body>
<h1>Display Output - JavaScript Tutorial</h1>
<script>
console.log("you can try to fix the bugs here..");
</script>
</body>
</html>
JavaScript Print
JavaScript does not have any print object or print methods.
window.print() method is used in the browser to print the content of the current window.
<!DOCTYPE html>
<html>
<body>
<h1>Follow Sagar InspireMe on YouTube Channel</h1>
<button onclick="window.print()">Print this page</button>
</body>
</html>
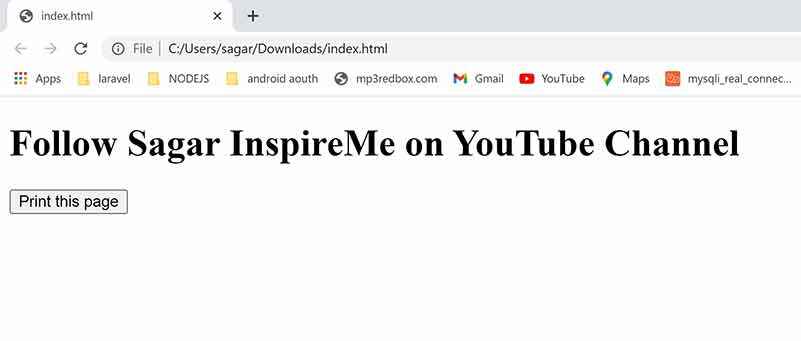
JavaScript Print Button using in HTML
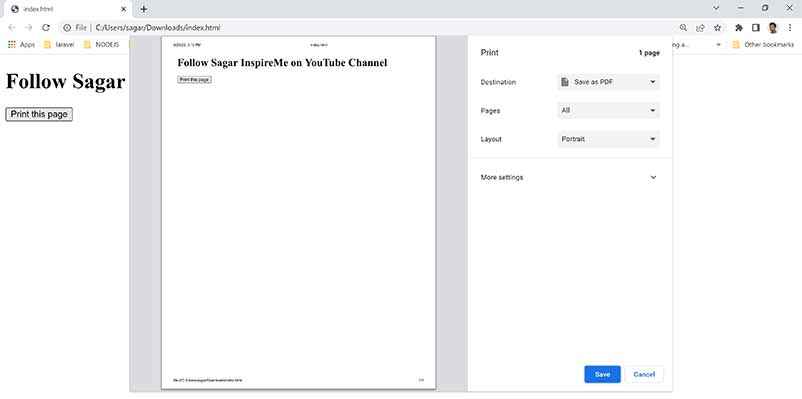
Clicked on JavaScript Print button, and displaying print page setting to save a file