How to invert (transpose or swap) the rows and columns of an HTML table?
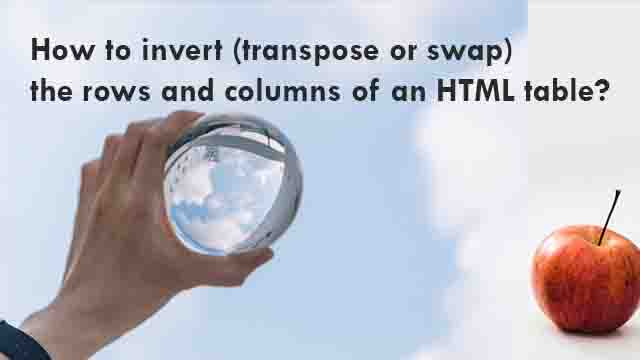
We are using J Query to make rows into a column in HTML table. You can use the code below to invert the rows and columns of an HTML table.
Solution: Using HTML, CSS and JQuery
<!-- HTML -->
<table>
<tr>
<td>1</td>
<td>4</td>
<td>7</td>
</tr>
<tr>
<td>2</td>
<td>5</td>
<td>8</td>
</tr>
<tr>
<td>3</td>
<td>6</td>
<td>9</td>
</tr>
</table>
<p><a href="#">Do it.</a></p>
<!-- JQUERY -->
<script>
$("a").click(function(){
$("table").each(function() {
var $this = $(this);
var newrows = [];
$this.find("tr").each(function(){
var i = 0;
$(this).find("td").each(function(){
i++;
if(newrows[i] === undefined) { newrows[i] = $("<tr></tr>"); }
newrows[i].append($(this));
});
});
$this.find("tr").remove();
$.each(newrows, function(){
$this.append(this);
});
});
return false;
});
</script>
CSS code:
td { padding:5px; border:1px solid #ccc;}
Source:jsfiddle
You can apply JavaScript to invert or transpose the rows and columns of an HTML table by doing what is described below:
- Using JavaScript, get the HTML table element.
- To save the transposed data, make a new, empty array.
- Retrieve the cells by iterating through all of the table's rows.
- Each cell's value should be iterated over and stored at the proper column and row index in the transposed array.
- Transpose the data to a new HTML table element before adding it.
- Substitute the transposed table for the original one.
Javascript Transponse Sample Code:
Example#1:
When a button is clicked, it transpose for one time only.
// Step 1: Retrieve the HTML table element
var table = document.getElementById('your-table-id');
// Step 2: Create an empty array for transposed data
var transposedData = [];
// Step 3: Iterate over rows
for (var i = 0; i < table.rows.length; i++) {
var row = table.rows[i];
var transposedRow = [];
// Step 4: Iterate over cells
for (var j = 0; j < row.cells.length; j++) {
var cell = row.cells[j];
transposedRow.push(cell.innerText || cell.textContent);
}
// Push the transposed row to the transposedData array
transposedData.push(transposedRow);
}
// Step 5: Create a new table for transposed data
var transposedTable = document.createElement('table');
// Iterate over the transposed data and create new rows and cells
for (var i = 0; i < transposedData[0].length; i++) {
var row = transposedTable.insertRow();
for (var j = 0; j < transposedData.length; j++) {
var cell = row.insertCell();
cell.textContent = transposedData[j][i];
}
}
// Step 6: Replace the original table with the transposed table
table.parentNode.replaceChild(transposedTable, table);
Here's an example of how you can implement the JavaScript code within an HTML document:
<!DOCTYPE html>
<html>
<head>
<title>Table Transposition</title>
<script>
function transposeTable() {
// Step 1: Retrieve the HTML table element
var table = document.getElementById('your-table-id');
// Step 2: Create an empty array for transposed data
var transposedData = [];
// Step 3: Iterate over rows
for (var i = 0; i < table.rows.length; i++) {
var row = table.rows[i];
var transposedRow = [];
// Step 4: Iterate over cells
for (var j = 0; j < row.cells.length; j++) {
var cell = row.cells[j];
transposedRow.push(cell.innerText || cell.textContent);
}
// Push the transposed row to the transposedData array
transposedData.push(transposedRow);
}
// Step 5: Create a new table for transposed data
var transposedTable = document.createElement('table');
// Iterate over the transposed data and create new rows and cells
for (var i = 0; i < transposedData[0].length; i++) {
var row = transposedTable.insertRow();
for (var j = 0; j < transposedData.length; j++) {
var cell = row.insertCell();
cell.textContent = transposedData[j][i];
}
}
// Step 6: Replace the original table with the transposed table
table.parentNode.replaceChild(transposedTable, table);
}
</script>
</head>
<body>
<table id="your-table-id">
<tr>
<td>A</td>
<td>B</td>
<td>C</td>
</tr>
<tr>
<td>1</td>
<td>2</td>
<td>3</td>
</tr>
<tr>
<td>X</td>
<td>Y</td>
<td>Z</td>
</tr>
</table>
<button onclick="transposeTable()">Transpose Table</button>
</body>
</html>
Example#2:
When you click the "Transpose Table" button, you may change the JavaScript code as needed to switch between the original and transposed tables:
var originalTable;
var transposedTable;
var isTransposed = false;
function transposeTable() {
// Retrieve the HTML table element
var table = document.getElementById('your-table-id');
// If the table is not transposed, create the transposed table
if (!isTransposed) {
// Create an empty array for transposed data
var transposedData = [];
// Iterate over rows
for (var i = 0; i < table.rows.length; i++) {
var row = table.rows[i];
var transposedRow = [];
// Iterate over cells
for (var j = 0; j < row.cells.length; j++) {
var cell = row.cells[j];
transposedRow.push(cell.innerText || cell.textContent);
}
// Push the transposed row to the transposedData array
transposedData.push(transposedRow);
}
// Create a new table for transposed data
transposedTable = document.createElement('table');
// Iterate over the transposed data and create new rows and cells
for (var i = 0; i < transposedData[0].length; i++) {
var row = transposedTable.insertRow();
for (var j = 0; j < transposedData.length; j++) {
var cell = row.insertCell();
cell.textContent = transposedData[j][i];
}
}
// Replace the original table with the transposed table
table.parentNode.replaceChild(transposedTable, table);
isTransposed = true;
} else { // If the table is already transposed, revert back to the original table
transposedTable.parentNode.replaceChild(originalTable, transposedTable);
isTransposed = false;
}
}
window.onload = function() {
// Store the original table when the page loads
originalTable = document.getElementById('your-table-id').cloneNode(true);
};
To toggle between the original table and the transposed table when clicking the "Transpose Table" button, you can implement the JavaScript code in your HTML template code as follows:
<!DOCTYPE html>
<html>
<head>
<title>Table Transposition</title>
<script>
var originalTable;
var transposedTable;
var isTransposed = false;
function transposeTable() {
// Retrieve the HTML table element
var table = document.getElementById('your-table-id');
// If the table is not transposed, create the transposed table
if (!isTransposed) {
// Create an empty array for transposed data
var transposedData = [];
// Iterate over rows
for (var i = 0; i < table.rows.length; i++) {
var row = table.rows[i];
var transposedRow = [];
// Iterate over cells
for (var j = 0; j < row.cells.length; j++) {
var cell = row.cells[j];
transposedRow.push(cell.innerText || cell.textContent);
}
// Push the transposed row to the transposedData array
transposedData.push(transposedRow);
}
// Create a new table for transposed data
transposedTable = document.createElement('table');
// Iterate over the transposed data and create new rows and cells
for (var i = 0; i < transposedData[0].length; i++) {
var row = transposedTable.insertRow();
for (var j = 0; j < transposedData.length; j++) {
var cell = row.insertCell();
cell.textContent = transposedData[j][i];
}
}
// Replace the original table with the transposed table
table.parentNode.replaceChild(transposedTable, table);
isTransposed = true;
} else { // If the table is already transposed, revert back to the original table
transposedTable.parentNode.replaceChild(originalTable, transposedTable);
isTransposed = false;
}
}
window.onload = function() {
// Store the original table when the page loads
originalTable = document.getElementById('your-table-id').cloneNode(true);
};
</script>
</head>
<body>
<table id="your-table-id">
<tr>
<td>A</td>
<td>B</td>
<td>C</td>
</tr>
<tr>
<td>1</td>
<td>2</td>
<td>3</td>
</tr>
<tr>
<td>X</td>
<td>Y</td>
<td>Z</td>
</tr>
</table>
<button onclick="transposeTable()">Transpose Table</button>
</body>
</html>
With this implementation, clicking the "Transpose Table" button toggles between the original table and the transposed table, allowing you to swap the rows and columns back and forth.