PHP Multidimensional Arrays - PHP Arrays
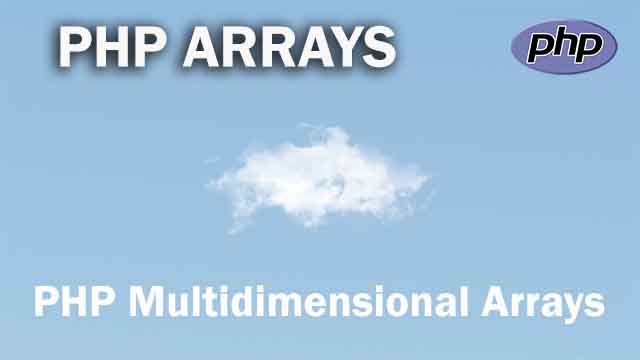
A multidimensional array is an array containing one or more arrays. PHP supports multidimensional arrays that are two, three, four, five, or more levels deep. However, arrays more than three levels deep are hard to manage for most people.
PHP Multidimensional array is used to store an array in contrast to constant values.
Multidimensional associative array is often used to store data in group relation.
Sometimes you want to store values with more than one key. For this, we have multidimensional arrays.
The below program demonstrate how to create a multidimensional associative array:
<?php
$languages = array();
$languages['Python'] = array(
"first_release" => "1991",
"latest_release" => "3.8.0",
"designed_by" => "Guido van Rossum",
"description" => array(
"extension" => ".py",
"typing_discipline" => "Duck, dynamic, gradual",
"license" => "Python Software Foundation License"
)
);
$languages['PHP'] = array(
"first_release" => "1995",
"latest_release" => "7.3.11",
"designed_by" => "Rasmus Lerdorf",
"description" => array(
"extension" => ".php",
"typing_discipline" => "Dynamic, weak",
"license" => "PHP License (most of Zend engine
under Zend Engine License)"
)
);
print_r($languages);
?>
Output:
Array
(
[Python] => Array
(
[first_release] => 1991
[latest_release] => 3.8.0
[designed_by] => Guido van Rossum
[description] => Array
(
[extension] => .py
[typing_discipline] => Duck, dynamic, gradual
[license] => Python Software Foundation License
)
)
[PHP] => Array
(
[first_release] => 1995
[latest_release] => 7.3.11
[designed_by] => Rasmus Lerdorf
[description] => Array
(
[extension] => .php
[typing_discipline] => Dynamic, weak
[license] => PHP License (most of Zend engine
under Zend Engine License)
)
)
)
Explanation: The parent indexes in the program above are Python and PHP. An array of sets of keys with fixed values is connected to the parent key. A different array containing the set of keys and constant values has been linked to the last key, which is the description of each parent key. Here, first release, latest release, designed by, and description are parent keys for Python and PHP, whereas extension, typing discipline, and license are parent keys for description.
Multidimensional arrays of two, three, four, five, or more levels of depth are supported by PHP. However, most individuals find it challenging to maintain arrays that are more than three levels deep.
The dimension of an array tells you how many indices are required to choose an element.
- To select an element from a two-dimensional array, you need two indices.
- To select an element from a three-dimensional array, you need three indices.
PHP - Two-dimensional Arrays
We can store the data in a two-dimensional array, like this:
$cars = array (
array("Audi","white",8),
array("BMW","blue",13),
array("Mercedes-Benz","golden",2),
array("Land Rover","black",15)
);
Code Example of two-dimensional arrays
<?php
$cars = array (
array("Audi","white",8),
array("BMW","blue",13),
array("Mercedes-Benz","golden",2),
array("Land Rover","black",15)
);
echo $cars[0][0].": Color: ".$cars[0][1].", sold: ".$cars[0][2].".<br>";
echo $cars[1][0].": Color: ".$cars[1][1].", sold: ".$cars[1][2].".<br>";
echo $cars[2][0].": Color: ".$cars[2][1].", sold: ".$cars[2][2].".<br>";
echo $cars[3][0].": Color: ".$cars[3][1].", sold: ".$cars[3][2].".<br>";
?>
Output:
Audi: Color: white, sold: 8.
BMW: Color: blue, sold: 13.
Mercedes-Benz: Color: golden, sold: 2.
Land Rover: Color: black, sold: 15.