Python Variables: Definition, Usage, and Examples
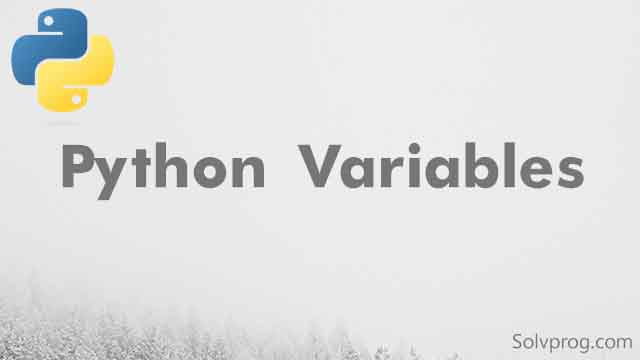
In Python, a variable is like a container that holds a value. You can put different types of values in the container, like words or numbers, and you can change the value whenever you want. Variables are important because they help you keep track of information in your program.
Python Variables
A variable in Python is a named container that holds a value. You can think of a variable as a label that refers to a specific value in memory.
When you create a variable in Python, you don't need to declare the type explicitly. The type of the variable is determined by the value that you assign to it. For example, if you assign a string to a variable, the variable will be of type string. If you assign an integer to a variable, the variable will be of type integer.
Here's an example of creating a variable in Python:
my_variable = "Hello, World!"
In this example, we are creating a variable named my_variable and assigning the string value "Hello, World!" to it.
You can also assign different types of values to the same variable. For example:
my_variable = 42 # integer value
my_variable = "Hello, World!" # string value
In this Python example, you can assign different types of values to the same variable. For example, you can assign an integer value to a variable and later assign a string value to the same variable. This is possible because Python variables are dynamically typed. This means that the type of a variable can change at any time.
When naming variables in Python, there are some rules to follow. Variable names must start with a letter or an underscore character, and can include letters, numbers, and underscores. Variable names are case-sensitive, which means that "myVariable" and "myvariable" are different names. It's also good practice to use descriptive variable names that indicate the purpose of the variable.
Overall, variables are an important concept in Python programming because they allow you to store and manipulate data values.
Python is a dynamically typed language, which means that you don't need to declare the type of a variable explicitly. The value assigned to a variable determines its type.
Here are some guidelines for naming variables in Python:
- Variable names must start with a letter or underscore character.
- The name can contain letters, numbers, and underscores.
- Variable names are case-sensitive.
- Avoid using reserved keywords (such as if, for, while, else, etc.) as variable names.
Here are some examples of valid variable names in Python:
my_variable
user_input
age
total_score
You can use variables in Python to store and manipulate data values, making it a powerful tool for programming.
Example Python Code: Variable Assignment
# Assigning an integer value to a variable
n = 500
# Printing the value of the variable
print(n)
# Reassigning the variable with a new value
n = 1000
# Printing the new value of the variable
print(n)
# Chained assignment
a = b = c = 500
print(a, b, c)
When you run this code, it will output:
500
1000
500 500 500
This demonstrates how variables can be assigned values and then used in statements or expressions. It also shows how variables can be reassigned with new values and how chained assignment can be used to assign the same value to multiple variables at once.
Variable Types in Python
Here is an example that demonstrates variable assignment and re-assignment with different data types:
# Assigning an integer value to a variable
x = 5
print(x)
# Re-assigning a string value to the same variable
x = "hello"
print(x)
# Re-assigning a boolean value to the same variable
x = True
print(x)
# Re-assigning a float value to the same variable
x = 3.14
print(x)
Output:
5
hello
True
3.14
As you can see, the same variable 'x' is assigned different data types (integer, string, boolean, and float) without any error.
Reserved Words (Keywords) in Python: Words You Can't Use as Variable Names
In Python, there are certain words that have special meanings and are reserved for use by the language itself. These words are called keywords or reserved words, and they cannot be used as variable names.
Here's a list of all the Python keywords as of Python 3.10:
False class from or None continue
finally import pass True def global
nonlocal raise and del if try
as elif in assert else is
with async for lambda return yield
await break except not while
If you try to use one of these keywords as a variable name, you'll get a syntax error. To avoid this, it's best to use descriptive variable names that indicate the purpose of the variable.
Here's an example of a syntax error that occurs when a reserved keyword is used as a variable name:
# Trying to use 'if' as a variable name - this will result in a syntax error
if = 10
When you try to run this code, you will get similar like this error message:
File "", line 2
if = 10
^
SyntaxError: invalid syntax
This error occurs because 'if' is a reserved keyword in Python and cannot be used as a variable name.
Conclusion
In conclusion, Python variables are symbolic names or labels that represent an object in memory. They are dynamically typed, which means their type can change at runtime. Variables must follow certain naming conventions and cannot use reserved keywords.
In Python, variables are essential for storing and manipulating data values. They allow programmers to create, access, and modify data objects, making it possible to write powerful and complex programs.
By understanding the basics of Python variables, you now have a solid foundation for learning more advanced topics such as data structures, control flow, and functions.