Python Operators with Examples
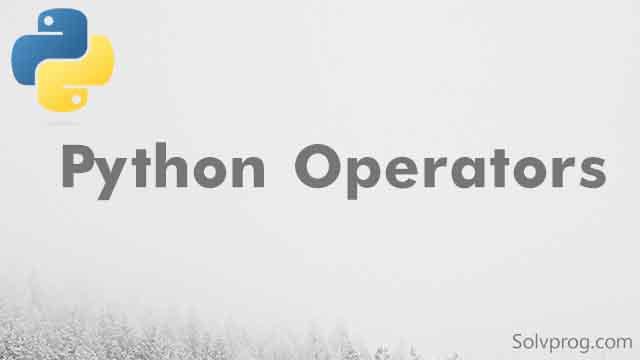
Python operators are symbols or keywords that allow you to perform operations on variables and values. There are various types of operators in Python, including arithmetic, comparison, assignment, logical, bitwise, identity, and membership operators. Understanding these operators is crucial in Python programming as they are used widely to perform various tasks. In this tutorial, we will provide an overview of Python operators and provide examples of their usage.
Python Operators
Python operators are special symbols or keywords that perform specific operations on one or more operands. In simple terms, operators are used to manipulate data values or variables in Python.
Python has a wide range of operators, including arithmetic, assignment, comparison, logical, bitwise, membership, and identity operators.
Arithmetic operators are used to perform mathematical operations such as addition, subtraction, multiplication, division, modulus, and exponentiation.
Assignment operators are used to assign values to variables, such as the equals sign (=) and the shorthand operators such as +=, -=, *=, and /=.
Comparison operators are used to compare two values and return a Boolean value of True or False. These operators include <, >, <=, >=, ==, and != .
Logical operators are used to combine multiple conditions and return a Boolean value. These operators include and, or, and not.
Bitwise operators are used to perform bit-level operations on integers, such as AND, OR, XOR, and NOT.
Membership operators are used to test if a sequence or collection contains a specified value. These operators include in and not in.
Identity operators are used to compare the memory location of two objects. These operators include is and is not.
In summary, Python operators are a fundamental concept in programming and are used commonly to perform various operations on data values and variables.
Arithmetic operators:
# Addition
a = 5 + 2
print(a) # Output: 7
# Subtraction
b = 8 - 3
print(b) # Output: 5
# Multiplication
c = 4 * 3
print(c) # Output: 12
# Division
d = 7 / 2
print(d) # Output: 3.5
# Modulus
e = 7 % 2
print(e) # Output: 1
# Exponentiation
f = 2 ** 3
print(f) # Output: 8
Comparison operators:
# Equal to
a = (5 == 5)
print(a) # Output: True
# Not equal to
b = (5 != 2)
print(b) # Output: True
# Greater than
c = (7 > 4)
print(c) # Output: True
# Less than
d = (3 < 6)
print(d) # Output: True
# Greater than or equal to
e = (6 >= 6)
print(e) # Output: True
# Less than or equal to
f = (2 <= 5)
print(f) # Output: True
Logical operators:
# And
a = (5 > 2) and (4 < 8)
print(a) # Output: True
# Or
b = (5 > 2) or (4 > 8)
print(b) # Output: True
# Not
c = not(5 > 2)
print(c) # Output: False
Assignment operators:
# Simple assignment
a = 5
print(a) # Output: 5
# Add and assign
a += 3
print(a) # Output: 8
# Subtract and assign
a -= 2
print(a) # Output: 6
# Multiply and assign
a *= 2
print(a) # Output: 12
# Divide and assign
a /= 3
print(a) # Output: 4.0
# Modulus and assign
a %= 2
print(a) # Output: 0.0
# Exponentiate and assign
a **= 3
print(a) # Output: 0.0
These are just a few examples of Python operators. Operators are a fundamental concept in programming and are used extensively to manipulate data and control program flow.
Basic concepts using operators:
# Assignment operator
x = 10
y = 5
# Arithmetic operators
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
print(x % y) # Modulus
# Comparison operators
print(x == y) # Equal to
print(x != y) # Not equal to
print(x > y) # Greater than
print(x < y) # Less than
print(x >= y) # Greater than or equal to
print(x <= y) # Less than or equal to
# Logical operators
print(x > 5 and y < 10) # Logical AND
print(x > 5 or y < 1) # Logical OR
print(not(x > 5 and y < 10)) # Logical NOT
# Bitwise operators
print(x & y) # Bitwise AND
print(x | y) # Bitwise OR
print(~x) # Bitwise NOT
print(x ^ y) # Bitwise XOR
print(x << 2) # Left shift
print(x >> 2) # Right shift
# Assignment operators
x += y # Equivalent to x = x + y
x -= y # Equivalent to x = x - y
x *= y # Equivalent to x = x * y
x /= y # Equivalent to x = x / y
x %= y # Equivalent to x = x % y
x //= y # Equivalent to x = x // y
x **= y # Equivalent to x = x ** y
x &= y # Equivalent to x = x & y
x |= y # Equivalent to x = x | y
x ^= y # Equivalent to x = x ^ y
x <<= y # Equivalent to x = x << y
x >>= y # Equivalent to x = x >> y
In this code, we have used various operators such as arithmetic, comparison, logical, bitwise, and assignment operators to perform operations on variables x and y. The comments above each line of code explain what the operator does.
Conclusion
In this tutorial, we covered the basics of Python operators, including arithmetic, comparison, assignment, logical, and bitwise operators. We also saw some examples of how to use these operators in Python code.
Understanding operators is essential in Python and any other programming language because they enable us to perform various operations on data values. By combining operators and operands, we can create expressions that accomplish a wide range of tasks.
It's important to keep in mind that operators have precedence rules, which determine the order in which operators are evaluated in an expression. We also need to be aware of the types of operands that operators work with, as they can sometimes behave differently depending on the data types.
Overall, operators are an essential concept in Python and are used extensively in programming to perform a wide range of operations on data values.