Python Functions with Examples
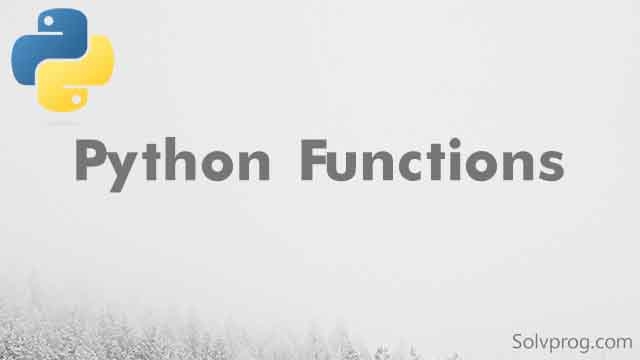
Functions are an essential aspect of any programming language. In Python, a function is a block of reusable code that performs a specific task. A function can take arguments (inputs), perform some operations, and return a result (output).
Python Functions
A function in Python is a block of organized, reusable code that performs a specific task. It takes input (arguments), performs some operations on it, and returns the output. Functions help break our program into smaller and modular chunks, making it more organized, efficient, and easy to understand and maintain.
In Python, we define a function using the "def" keyword followed by the function name, arguments (if any), and a colon. The function body is indented and contains the statements that define the function's behavior.
Here's an example function definition in Python:
def greet(name):
"""
This function greets the person passed in as a parameter
"""
print("Hello, " + name + ". How are you doing today?")
In this example, we define a function called "greet" that takes a single argument, "name". The function body contains a single statement that prints a greeting message using the value of the "name" argument.
Note that the function definition starts with a docstring, which is a string literal used to document the function. It is enclosed in triple quotes and is optional but highly recommended for documenting your code.
Example1:
Here's an example of a simple function that takes two arguments and returns their sum:
def add_numbers(a, b):
result = a + b
return result
To call this function, we simply pass in the arguments and assign the result to a variable:
sum = add_numbers(3, 5)
print(sum) # Output: 8
Example2:
In Python, a function can also return multiple values, which are typically returned as a tuple:
def calculate_stats(numbers):
total = sum(numbers)
count = len(numbers)
mean = total / count
return total, count, mean
result = calculate_stats([1, 2, 3, 4, 5])
print(result) # Output: (15, 5, 3.0)
Example3:
Functions can also have default argument values, which are used when an argument is not explicitly provided:
def greet(name='World'):
print(f'Hello, {name}!')
greet() # Output: Hello, World!
greet('Alice') # Output: Hello, Alice!
Example4:
Additionally, Python supports lambda functions, which are small anonymous functions typically used for simple operations. Here's an example:
double = lambda x: x * 2
result = double(5)
print(result) # Output: 10
Overall, functions are a powerful tool in Python for structuring code and making it more reusable. By using functions, we can break down complex tasks into smaller, more manageable pieces of code.
Challenging Python Function Example with Explanation
Here's an example function that could be challenging but also helpful for learning Python:
def calculate_factorial(n):
"""
This function takes a non-negative integer n as input and returns the factorial of n.
If n is negative, it raises a ValueError.
"""
if n < 0:
raise ValueError("Input must be non-negative")
elif n == 0:
return 1
else:
return n * calculate_factorial(n-1)
This function calculates the factorial of a non-negative integer using recursion. The factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. For example, 5! = 5 x 4 x 3 x 2 x 1 = 120.
To calculate the factorial of n, the function checks if n is negative, in which case it raises a ValueError. If n is zero, it returns 1 (since 0! = 1 by definition). Otherwise, it calculates the factorial of n-1 recursively and multiplies it by n to obtain the factorial of n.
This function demonstrates several important concepts in Python, including function definition, input validation, recursion, and conditional statements.
To display the output of the calculate_factorial function, you can call it with an argument and print the result. Here's an example:
# Call the function and store the result in a variable
result = calculate_factorial(5)
# Print the result
print(result)
This will output the factorial of 5, which is 120:
120
In conclusion, functions are an essential aspect of programming in Python. They help in organizing code and making it reusable. Functions in Python are defined using the def keyword followed by the function name and parameters. They can be called multiple times in the program, making code more efficient and concise. In this tutorial, we covered the basics of Python functions, including defining functions, passing arguments, and returning values, and we also gave an example of a function that calculates the factorial of a number. With this knowledge, you should now be able to write and use functions in your Python programs.