Python Lists, Tuples, and Dictionaries with Examples
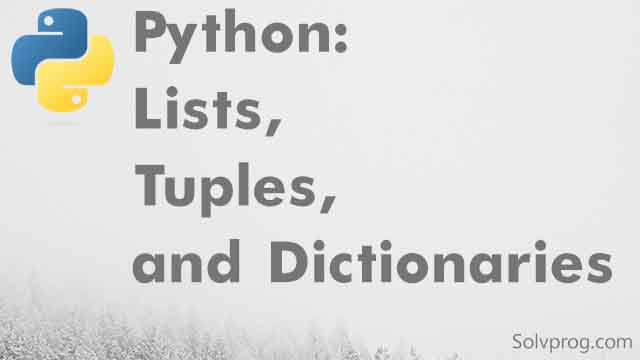
Python provides several data structures to store collections of data. Three commonly used data structures in Python are lists, tuples, and dictionaries.
Python Lists, Tuples, and Dictionaries
Python has three built-in data structures to store collections of data: lists, tuples, and dictionaries.
A list is an ordered collection of items, which can be of different data types. Lists are mutable, meaning their values can be changed.
A tuple. is also an ordered collection of items, but unlike lists, tuples are immutable, meaning their values cannot be changed.
A dictionary is an unordered collection of key-value pairs. Each key in a dictionary is unique and associated with a value.
Here are some examples:
- A list of fruits: ['apple', 'banana', 'orange']
- A tuple of coordinates: (3, 4)
- A dictionary of ages: {'Alice': 30, 'Bob': 25, 'Charlie': 35}
Lists, tuples, and dictionaries can be accessed and manipulated using various methods and operators provided by Python.
Lists in Python with example:
In this example, we create a list of numbers and demonstrate various list operations such as accessing elements by index, modifying elements, adding elements, removing elements, and iterating over the list.
# create a list of numbers
numbers = [1, 2, 3, 4, 5]
# access elements in the list by index
print(numbers[0]) # output: 1
print(numbers[2]) # output: 3
# modify elements in the list
numbers[1] = 7
print(numbers) # output: [1, 7, 3, 4, 5]
# add elements to the list
numbers.append(6)
print(numbers) # output: [1, 7, 3, 4, 5, 6]
# remove elements from the list
numbers.remove(3)
print(numbers) # output: [1, 7, 4, 5, 6]
# iterate over the list
for number in numbers:
print(number)
# output:
# 1
# 7
# 4
# 5
# 6
Tuples in Python with example:
# Creating a tuple
my_tuple = (1, 2, 3, 4, 5)
# Accessing elements of a tuple
print(my_tuple[0]) # Output: 1
print(my_tuple[3]) # Output: 4
# Slicing a tuple
print(my_tuple[1:4]) # Output: (2, 3, 4)
# Trying to modify a tuple (This will result in a TypeError)
my_tuple[2] = 6
In the example above, we create a tuple my_tuple with 5 elements. We then access elements of the tuple using indexing and slicing. However, since tuples are immutable, we cannot modify its elements directly. Any attempt to do so will result in a TypeError.
Dictionary in Python with example:
# Creating a dictionary to store information about a person
person = {"name": "John", "age": 30, "city": "New York"}
# Accessing values in the dictionary
print("Name:", person["name"])
print("Age:", person["age"])
print("City:", person["city"])
# Adding a new key-value pair to the dictionary
person["occupation"] = "Engineer"
# Updating the value of an existing key
person["age"] = 35
# Deleting a key-value pair from the dictionary
del person["city"]
# Iterating over the keys and values in the dictionary
for key, value in person.items():
print(key + ":", value)
In this example, we create a dictionary person that stores information about a person. We access values in the dictionary using their keys, and can add, update, or delete key-value pairs as needed. We can also iterate over the keys and values in the dictionary using a for loop and the items() method.
- Lists, tuples, and dictionaries are three different types of collection data structures in Python.
- Lists are created by enclosing a sequence of items in square brackets ([]), and can contain elements of different data types.
- Tuples are created by enclosing a sequence of items in parentheses (()), and are similar to lists but are immutable (i.e., their values cannot be changed after creation).
- Dictionaries are created by enclosing a set of key-value pairs in curly braces ({}), and are used to store data in a way that allows fast lookup based on a key.
- Lists and tuples are ordered, meaning that the items in them are indexed and can be accessed by their position in the sequence.
- Dictionaries are unordered, but their key-value pairs allow for efficient lookup and retrieval of data.
- Examples of using these data structures include creating a list of names, creating a tuple of coordinates, and creating a dictionary of customer information.
In conclusion, Python offers several built-in data structures such as lists, tuples, and dictionaries that allow you to store and manipulate data in different ways depending on your needs. Lists are mutable and can be modified, while tuples are immutable and cannot be modified after creation. Dictionaries allow you to store key-value pairs and retrieve values using their corresponding keys. By understanding these data structures and their respective methods and functions, you can effectively work with and manipulate data in your Python programs.