Python File Handling
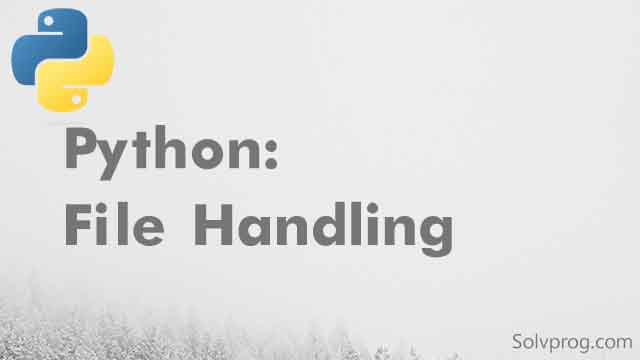
Python file handling refers to the process of working with files in a program. It allows you to read data from or write data to external files. Python provides built-in functions to open, read, write, and close files. To use file handling in Python, you need to open a file using the open() function, perform the desired operation using appropriate methods, and close the file using the close() method. By using file handling, you can store and retrieve data from files, which can be useful for saving program data, working with external data sources, or creating output files.
Python File Handling
Python file handling refers to the process of working with files in a program. Files can be used to store data, read data from an external source, or write data to an external destination. Python provides built-in functions for opening, reading, writing, and closing files, making it easy to work with files in a program.
To work with a file in Python, you need to perform the following steps:
1. Open the file using the open() function, which takes two parameters: the file name and the mode (read, write, or append). The function returns a file object that you can use to read from or write to the file.
2. Read or write data to the file using the appropriate method of the file object. For example, to read the contents of a file, you can use the read() method, and to write to a file, you can use the write() method.
3. Close the file using the close() method when you are finished working with it. This is an important step, as it frees up system resources and ensures that all data is written to the file before it is closed.
Here is an example code that explains very well how to read data from a file in Python:
# Open the file in read mode
file = open('example.txt', 'r')
# Read the contents of the file
contents = file.read()
# Print the contents of the file
print(contents)
# Close the file
file.close()
In this example, we use the open() function to open a file called example.txt in read mode. We then use the read() method to read the contents of the file and store them in a variable called contents. Finally, we print the contents of the file and close the file using the close() method.
Python also provides other file modes such as write and append. Here is an example code that demonstrates how to write data to a file:
# Open the file in write mode
file = open('example.txt', 'w')
# Write some data to the file
file.write('Hello, world!')
# Close the file
file.close()
In this example, we use the open() function to open a file called example.txt in write mode. We then use the write() method to write the string "Hello, world!" to the file. Finally, we close the file using the close() method.
Overall, Python file handling is a powerful feature that allows you to work with external files in your programs. Whether you are reading from a file, writing to a file, or both, Python provides the necessary tools to make file handling easy and efficient.
Example code that explain file handling in Python by reading data from a file and writing data to another file:
# Open the input file in read mode
with open('input.txt', 'r') as input_file:
# Read the contents of the file
data = input_file.read()
# Modify the data
modified_data = data.upper()
# Open the output file in write mode
with open('output.txt', 'w') as output_file:
# Write the modified data to the file
output_file.write(modified_data)
Here's a step-by-step explanation of the code:
- Open the input file called input.txt in read mode using the with statement. This ensures that the file is automatically closed when we are done with it.
- Use the read() method to read the contents of the file and store them in a variable called data.
- Modify the data by converting it to uppercase using the upper() method.
- Open the output file called output.txt in write mode using the with statement.
- This ensures that the file is automatically closed when we are done with it.
- Use the write() method to write the modified data to the file.
- Once the with block is exited, the output file is automatically closed.
Overall, the code reads the contents of an input file, modifies the data, and writes it to an output file. The with statement is used to safely and efficiently handle file I/O, ensuring that the files are properly opened and closed.
In summary, file handling in Python involves working with external files within a program. Python provides built-in functions and methods to open, read, write, and close files. The with statement is commonly used for safer and more efficient file handling, as it automatically closes the file when the block of code is exited. File handling in Python can be used for a variety of purposes, such as storing program data, reading from and writing to external data sources, and creating output files.