Python Classes/Objects with Examples
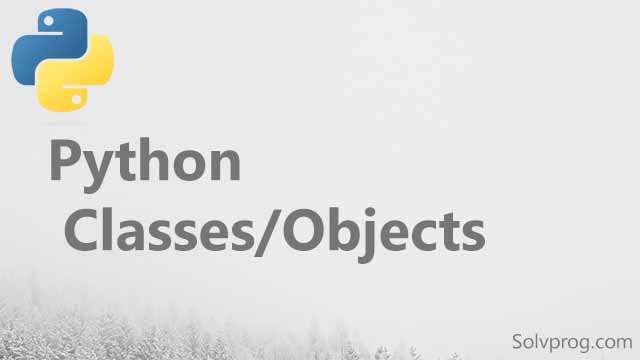
Python is an object-oriented programming language, which means that everything in Python is an object. An object is a representation of a real-world entity that has properties and behaviors. In Python, a class is a blueprint or a template for creating objects that share similar properties and behaviors. It's like a factory that produces objects with predefined characteristics.
Python Object-Oriented Programming (OOP) is a paradigm in which we define classes to represent objects and their properties and methods. A class is a blueprint for creating objects, which are instances of that class. In Python, we define classes using the class keyword.
1. Class
Here is the basic syntax for defining a class in Python:
class ClassName:
# class attributes
# methods
- In the above syntax, we start with the class keyword, followed by the name of the class (in CamelCase convention). The class definition can contain class attributes (variables) and methods.
- A class can have attributes and methods. Attributes are properties that define the state of an object, while methods are functions that define the behavior of the object.
Here's an example of a simple class with an attribute and a method:
class Person:
def __init__(self, name):
self.name = name
def say_hello(self):
print(f"Hello, my name is {self.name}")
- We define a class called "Person" with an attribute called "name".
- We initialize the "name" attribute using the __init__ method, which is called when a new instance of the class is created.
- The __init__ method takes two arguments: "self" (which refers to the instance of the class) and "name" (which is used to initialize the "name" attribute).
- We define a method called "say_hello" that prints a greeting message that includes the person's name.
- The "say_hello" method takes one argument: "self" (which refers to the instance of the class).
- We create a new instance of the "Person" class named "person1" and initialize its "name" attribute to "John".
- We call the "say_hello" method on the "person1" object, which prints a greeting message that includes the person's name.
Output: here's an example of how you can use this class to create an instance of a person:
person = Person("John")
person.say_hello() # Output: Hello, my name is John
Example 2:
We can also define attributes and methods inside the class to give the instances properties and behaviors. Here's an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print("Hello, my name is {} and I'm {} years old.".format(self.name, self.age))
In this example, we create a class named "Person" with two attributes: "name" and "age". We initialize these attributes using the __init__ method, which is called when an instance is created. Additionally, we define a method called "say_hello" that prints a greeting message including the person's name and age.
Output: we can create instances of this class and access their attributes and methods like this:
person1 = Person("John", 25)
person2 = Person("Jane", 30)
print(person1.name) # Output: John
print(person2.age) # Output: 30
person1.say_hello() # Output: Hello, my name is John and I'm 25 years old.
person2.say_hello() # Output: Hello, my name is Jane and I'm 30 years old.
In the above example, a class is a blueprint for creating objects that share similar properties and behaviors. It's defined using the class keyword, and can contain attributes and methods that define the state and behavior of the objects created from the class.
Example 3:
here's an example of Python Object-Oriented Programming (OOP) with a simple class:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def get_description(self):
return f"{self.year} {self.make} {self.model}"
def read_odometer(self):
return f"This car has {self.odometer_reading} miles on it."
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
- We define a class called "Car".
- The __init__ method is a constructor that initializes the make, model, year, and odometer_reading attributes of the class.
- The get_description method returns a formatted string with the car's make, model, and year.
- The read_odometer method returns the number of miles on the car's odometer.
- The update_odometer method updates the car's odometer reading, but only if the new reading is greater than or equal to the previous reading.
- The increment_odometer method increments the odometer reading by a certain number of miles.
Output: here's an example of how you can use this class to create an instance of a car:
my_car = Car("Toyota", "Corolla", 2020)
print(my_car.get_description()) # Output: 2020 Toyota Corolla
my_car.update_odometer(10000)
print(my_car.read_odometer()) # Output: This car has 10000 miles on it.
my_car.increment_odometer(500)
print(my_car.read_odometer()) # Output: This car has 10500 miles on it.
In the example, we create a car instance with make "Toyota", model "Corolla", and year "2020". We print the car's description using the get_description method. Then, we update the odometer reading to 10,000 using the update_odometer method and print the odometer reading using the read_odometer method. Finally, we increment the odometer reading by 500 using the increment_odometer method and print the odometer reading again.
2. Objects
In Python, objects are instances of classes. They have properties (attributes) and methods, just like the classes they are created from. We create objects by creating instances of classes using the constructor method __init__() to initialize their attributes. Here's an example:
# Define a class called "Person"
class Person:
# Define the __init__ method to initialize the object's attributes
def __init__(self, name, age):
self.name = name
self.age = age
# Create an instance of the Person class
my_person = Person("John", 30)
# Access the object's attributes
print(my_person.name)
print(my_person.age)
In this example, we define a Person class with name and age attributes, and an __init__() method to initialize them. We create an instance of the Person class called my_person with the name "John" and age "30". We access the name and age attributes of the my_person object using dot notation and print their values.
Example
here's an example of Python OOPs objects:
# Define a class called "Dog"
class Dog:
# Define the __init__ method to initialize the object's attributes
def __init__(self, name, age):
self.name = name
self.age = age
# Define a method called "bark"
def bark(self):
print(f"{self.name} barks!")
# Create an instance of the Dog class
my_dog = Dog("Fido", 3)
# Call the bark method on the my_dog instance
my_dog.bark()
In the example above:
- We define a class called "Dog" with two attributes: "name" and "age".
- We initialize these attributes using the __init__ method, which is called when an instance of the class is created.
- We define a method called "bark" that prints a message saying that the dog is barking.
- We create an instance of the "Dog" class called "my_dog" with the name "Fido" and age "3".
- We call the "bark" method on the "my_dog" object, which prints a message saying that "Fido" is barking.
The output of the example code will be:
Fido barks!
This is because we create a Dog object called my_dog with the name "Fido" and age "3", and then call the bark() method on that object. The bark() method prints a message saying "Fido barks!", which is the output we see.