Inheritance in Python
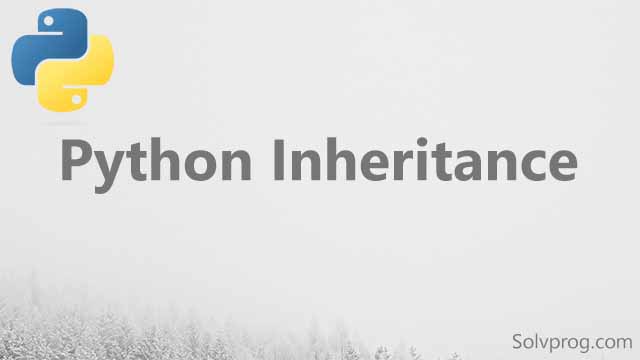
Inheritance is a concept in object-oriented programming (OOP) that allows one class to inherit properties and methods from another class. The class that is being inherited from is called the "parent" or "superclass", and the class that is inheriting is called the "child" or "subclass".
In Python, we can create a subclass by defining a new class and specifying the parent class in parentheses after the class name. The subclass will automatically inherit all of the properties and methods of the parent class. The subclass can also override or add new properties and methods as needed.
Here's an example:
# Define a parent class called "Animal"
class Animal:
def __init__(self, name):
self.name = name
def eat(self):
print("The animal is eating...")
# Define a subclass called "Dog" that inherits from "Animal"
class Dog(Animal):
def bark(self):
print("Woof! Woof!")
# Create an instance of the "Dog" class
my_dog = Dog("Fido")
# Access the object's attributes and call its methods
print(my_dog.name)
my_dog.eat()
my_dog.bark()
In this example of Inhritance using Python OOPs, we define a parent class called "Animal" and a subclass called "Dog" that inherits from "Animal". We create an instance of the Dog class called my_dog and access its attributes and call its methods. The Dog class has access to the eat() method defined in the Animal class, and it also has an additional method called bark().
Example 2
Here's an example of inheritance in Python using recipe classes.
class Recipe:
def __init__(self, name, ingredients, instructions):
self.name = name
self.ingredients = ingredients
self.instructions = instructions
def print_recipe(self):
print(f"{self.name}:")
print("Ingredients:")
for ingredient in self.ingredients:
print(f"- {ingredient}")
print("Instructions:")
for instruction in self.instructions:
print(f"- {instruction}")
print("\n")
class DessertRecipe(Recipe):
def __init__(self, name, ingredients, instructions, dessert_type):
super().__init__(name, ingredients, instructions)
self.dessert_type = dessert_type
def print_recipe(self):
super().print_recipe()
print(f"Dessert Type: {self.dessert_type}\n")
class SoupRecipe(Recipe):
def __init__(self, name, ingredients, instructions, soup_type):
super().__init__(name, ingredients, instructions)
self.soup_type = soup_type
def print_recipe(self):
super().print_recipe()
print(f"Soup Type: {self.soup_type}\n")
In this example, we create a Recipe class with an init() method to initialize the recipe attributes and a print_recipe() method to print the recipe. We then create two subclasses called DessertRecipe and SoupRecipe that inherit from the Recipe class. Each subclass has an additional attribute and a print_recipe() method that calls the parent class's print_recipe() method and then prints out the additional attributes specific to the subclass. This allows us to reuse code from the parent class and customize it for each subclass.
Here's an example of how we can create instances of these classes and call their methods:
chocolate_cake = DessertRecipe("Chocolate Cake", ["flour", "sugar", "eggs", "cocoa powder"], ["Mix ingredients", "Bake at 350 degrees"], "Cake")
chicken_noodle_soup = SoupRecipe("Chicken Noodle Soup", ["chicken broth", "noodles", "chicken", "carrots", "celery"], ["Boil broth", "Add noodles and vegetables", "Simmer until cooked"], "Noodle")
chocolate_cake.print_recipe()
chicken_noodle_soup.print_recipe()
When we run this code, it will print out the recipes for chocolate cake and chicken noodle soup, with the dessert type for the chocolate cake and soup type for the chicken noodle soup. Since the print_recipe() method is defined in the Recipe class and overridden in the subclasses, each subclass's print_recipe() method will call the parent class's print_recipe() method first and then print out additional information specific to that subclass.
Output: The output of the example code would be:
--- Chocolate Cake Recipe ---
Ingredients: flour, sugar, eggs, cocoa powder
Instructions:
1. Mix ingredients
2. Bake at 350 degrees
Dessert Type: Cake
--- Chicken Noodle Soup Recipe ---
Ingredients: chicken broth, noodles, chicken, carrots, celery
Instructions:
1. Boil broth
2. Add noodles and vegetables
3. Simmer until cooked
Soup Type: Noodle
The output displays the recipe details for both the Chocolate Cake and Chicken Noodle Soup objects using their respective print_recipe() methods. The output includes the recipe name, list of ingredients, list of instructions, and additional attributes specific to each subclass (DessertRecipe and SoupRecipe).
Inheritance is a fundamental concept in object-oriented programming, and it allows for the creation of subclasses that inherit attributes and methods from a parent class. This helps to reduce code duplication and allows for the creation of specialized classes that have additional attributes and methods. In Python, we use the keyword class to define a class, and the keyword super() to call the parent class's methods. Inheritance is a powerful tool for creating complex programs that are easy to read and maintain.