Encapsulation in Python
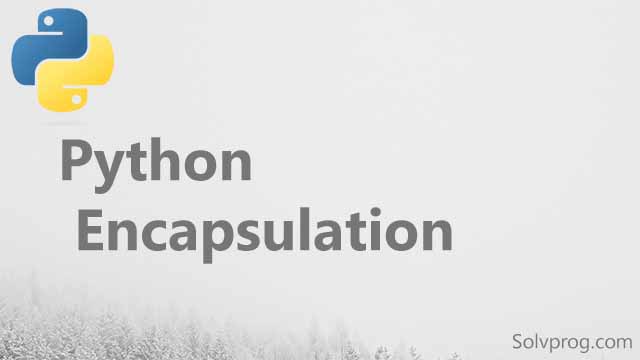
Encapsulation is a fundamental concept in object-oriented programming that involves bundling data and methods that operate on that data within a single unit or class. The main purpose of encapsulation is to hide the implementation details of an object from the outside world, and to protect the integrity and security of the data stored in that object.
Encapsulation
Encapsulation is the process of bundling data and methods together within a single unit or class, and controlling access to them through access modifiers. This helps to hide the implementation details of an object from the outside world and ensure that the data is used in a safe and consistent way. In Python, access modifiers such as public, private, and protected are used to control access to data and methods within a class.
In Python, encapsulation is achieved through the use of access modifiers such as public, private, and protected. These access modifiers determine whether the data and methods of an object can be accessed from outside the class or not.
Public members are those that are accessible from anywhere in the program, including from outside the class. Private members, on the other hand, are only accessible from within the class, and are denoted by using the double underscore (__) prefix. Protected members are those that can be accessed from within the class and its subclasses, and are denoted by using a single underscore (_) prefix.
By encapsulating data and methods within a class and controlling access to them through access modifiers, we can ensure that the data is used in a safe and consistent way, and that the implementation details of the object are hidden from the outside world.
Example: encapsulation using all three access modifiers: public, private, and protected:
class Car:
def __init__(self, make, model, year):
self.make = make # public attribute
self._model = model # protected attribute
self.__year = year # private attribute
def display(self):
print("Make:", self.make)
print("Model:", self._model)
print("Year:", self.__year)
def change_year(self, new_year):
self.__year = new_year
def __calculate_mileage(self, distance, fuel):
return distance/fuel
def get_mileage(self, distance, fuel):
return self.__calculate_mileage(distance, fuel)
car = Car("Honda", "Civic", 2021)
# Accessing public member outside the class
print(car.make)
# Accessing protected member outside the class
print(car._model)
# Accessing private member outside the class raises an AttributeError
print(car.__year)
# Accessing private member using name mangling
print(car._Car__year)
# Changing the value of private member using public method
car.change_year(2022)
car.display()
# Accessing private method using public method
mileage = car.get_mileage(200, 10)
print("Mileage:", mileage)
The output of the code will be:
Honda
Civic
AttributeError: 'Car' object has no attribute '__year'
2021
Make: Honda
Model: Civic
Year: 2022
Mileage: 20.0
Explanation:
- We create a Car object with make = "Honda", model = "Civic", and year = 2021.
- We print the value of the public attribute make using the dot notation, and get "Honda".
- We print the value of the protected attribute _model using the dot notation, and get "Civic".
- We try to print the value of the private attribute __year using the dot notation, which raises an AttributeError since private attributes cannot be accessed from outside the class.
- We use name mangling to access the private attribute __year, which returns its value of 2021.
- We use the public method change_year() to change the value of the private attribute __year to 2022, and then call the display() method to print the new value of year.
- We use the public method get_mileage() to calculate the mileage of the car given the distance travelled and fuel used, and then print the result of 20.0.
Encapsulation is an important concept in object-oriented programming that helps in creating robust, secure, and reusable code. By encapsulating data and methods within a class, we can control access to them from outside the class, thus ensuring data integrity and preventing accidental modification of critical data.
Encapsulation also helps in achieving abstraction, as the user of a class does not need to know the implementation details of the class. They can simply use the public interface of the class to perform their desired actions, without worrying about how the class works internally.
In Python, encapsulation is achieved through the use of access modifiers, such as public, private, and protected attributes and methods. Public attributes and methods can be accessed from anywhere outside the class, protected attributes and methods can only be accessed from within the class and its subclasses, and private attributes and methods can only be accessed from within the class itself.
Overall, encapsulation is an essential concept in object-oriented programming that helps in creating code that is secure, maintainable, and reusable.