__init__ in Python | Python __init__() Function | What is __init__ in Python?
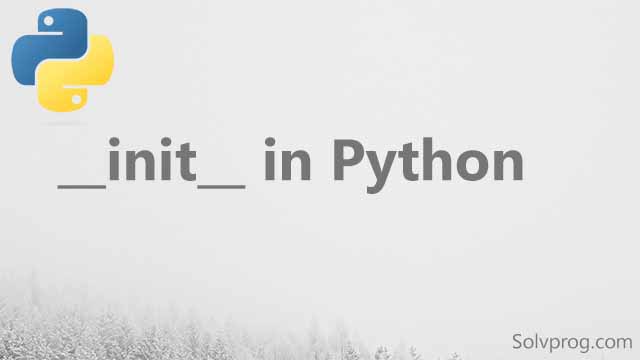
The __init__ method in Python is a special method that is used to initialize the object's attributes and perform any necessary setup when the object is created. It is called automatically every time an object is created from a class. Think of it like a constructor in other programming languages.
Python __init__()
In Python, __init__() is a special method (function) that is called when an object of a class is created. It is commonly used to initialize the attributes of the object. The __init__() method takes at least one argument, which is self, and it can take additional arguments depending on the requirements of the class.
Imagine you're running a pizza shop. Your pizza chef is like a class, and each pizza they make is like an object. Now, when your chef starts making a pizza, they need to know what kind of pizza it is (cheese, pepperoni, etc.), how big it should be, and any other special instructions from the customer. This is like the __init__() method in Python classes.
Just like your chef needs to know the pizza details every time they make a new pizza, the __init__() method is called every time an object is created from a class. It lets the class set up the object's initial state, or in other words, initialize its attributes.
And just like your chef wouldn't use this information for anything other than making the pizza, the __init__() method serves no other purpose within a class. It is a special method used exclusively for initializing object attributes.
So, think of the __init__() method as your chef's instructions for making a pizza. Every time a new pizza is made (i.e., a new object is created), the instructions are used to set up the pizza just the way the customer wants it.
What is __init__ in Python?
- The __init__() method is a special function that is called automatically when an object is created from a class.
- It is used to initialize the attributes of the object and set its initial state.
- The __init__() method takes one mandatory parameter, which is usually called self. This parameter refers to the object being created and allows you to access its attributes and methods.
- The self parameter is followed by any additional parameters that you want to use to initialize the object's attributes.
- The attributes of an object are usually defined as instance variables within the __init__() method. These variables are specific to each instance of the class and can be accessed and modified using the self parameter.
- The __init__() method is not required in a class, but it is considered good practice to use it to set up the object's initial state.
- If you don't define an __init__() method, Python will automatically create a default one for you that does nothing.
- The __init__() method is only used within classes and serves no other purpose. It's like a tool that only works when you're building an object.
Example Code: A Sample class with init method
Example 1
class Person:
def __init__(self, name, age, occupation):
self.name = name
self.age = age
self.occupation = occupation
def introduce(self):
print("Hi, my name is", self.name + ",", "I am", self.age, "years old and I work as a", self.occupation)
p1 = Person('Alice', 25, 'Software Engineer')
p2 = Person('Bob', 30, 'Data Scientist')
p1.introduce() # Output: Hi, my name is Alice, I am 25 years old and I work as a Software Engineer
p2.introduce() # Output: Hi, my name is Bob, I am 30 years old and I work as a Data Scientist
In this example, we have a Person class with an __init__() method that takes in name, age, and occupation as parameters, and sets them as attributes of the object. We also have a method called introduce() which prints out the person's name, age, and occupation.
We create two instances of the Person class called p1 and p2, and call the introduce() method on both instances to introduce them. The output will show the name, age, and occupation of each person.
Output:
Hi, my name is Alice, I am 25 years old and I work as a Software Engineer
Hi, my name is Bob, I am 30 years old and I work as a Data Scientist
Example 2
Here's an example of using the __init__() method in a blog class:
class Blog:
def __init__(self, title, content, author):
self.title = title
self.content = content
self.author = author
self.likes = 0
def like(self):
self.likes += 1
blog1 = Blog("My First Blog", "This is my first blog post!", "John Doe")
print(blog1.title) # Output: My First Blog
print(blog1.content) # Output: This is my first blog post!
print(blog1.author) # Output: John Doe
print(blog1.likes) # Output: 0
blog1.like()
print(blog1.likes) # Output: 1
In this example, we define a Blog class that has four attributes: title, content, author, and likes.
We use the __init__() method to initialize these attributes when we create a new Blog object. The __init__() method takes three parameters: title, content, and author. We use these parameters to set the initial values of the object's attributes.
We also set the likes attribute to 0 by default, since a new blog post would not have any likes yet.
We define a like() method that increments the likes attribute by 1 each time it is called.
Finally, we create a new Blog object called blog1 with the title "My First Blog", content "This is my first blog post!", and author "John Doe".
We print out the values of blog1's attributes using dot notation, and then call the like() method to increment the likes attribute. We print out the new value of blog1's likes attribute to confirm that it has been incremented correctly.
Output:
My First Blog
This is my first blog post!
John Doe
0
1
Example 3
Here's an example of using __init__ with inheritance:
class Animal:
def __init__(self, name):
self.name = name
print("An animal named", self.name, "has been created.")
def sound(self):
print("This animal makes a sound.")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
print("A dog of breed", self.breed, "has been created.")
def sound(self):
print("The dog barks.")
class Cat(Animal):
def __init__(self, name, color):
super().__init__(name)
self.color = color
print("A cat with color", self.color, "has been created.")
def sound(self):
print("The cat meows.")
my_dog = Dog("Fido", "Golden Retriever")
my_cat = Cat("Fluffy", "Gray")
my_dog.sound() # Output: The dog barks.
my_cat.sound() # Output: The cat meows.
This example demonstrates inheritance in Python using an Animal class with an init() method and a sound() method. The Dog and Cat subclasses inherit from the Animal class and override the sound() method with their own specific sounds. The subclasses have their own init() method that calls the parent class's init() method and sets their own attributes. Finally, we create instances of both subclasses and call their respective sound() methods to hear their specific sounds.
Output
The dog barks
The cat meows
Conclusion: __init__ method in Python
- The init method is a special method in Python.
- It is called every time an object is created from a class.
- It lets the class initialize the object's attributes and perform any necessary setup when the object is being created.
- The init method is commonly used to assign values to object properties or perform other operations that are necessary during object creation.
- It is similar to a constructor in other object-oriented programming languages.
- Understanding the init method is important in Python programming, as it is a fundamental concept that is used frequently in creating and working with classes and objects.