*args and **kwargs in Python
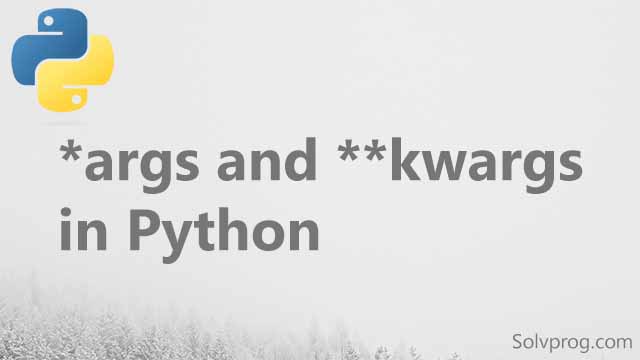
In Python, *args and **kwargs are used to pass a variable number of arguments to a function. It means that with *args and **kwargs, you can define a function that can accept any number of arguments, which can be either positional or keyword arguments. This makes the function more flexible and versatile, and allows you to write code that can handle a wide range of input.
In Python, there are two special symbols used for passing arguments:
*args: This is used to pass a variable number of non-keyword arguments (positional arguments) to a function. The * symbol before args tells Python to pack all the positional arguments into a tuple.
**kwargs: This is used to pass a variable number of keyword arguments to a function. The ** symbol before kwargs tells Python to pack all the keyword arguments into a dictionary.
So, you can think of *args as a way to pass a list of values to a function, and **kwargs as a way to pass a dictionary of values to a function.
*args Example
def my_function(*args):
for arg in args:
print(arg)
my_function("Hello", "world", "how", "are", "you")
Output:
Hello
world
how
are
you
In this example, the my_function() takes a variable number of positional arguments using *args. The for loop is used to iterate over the arguments and print them out.
**kwargs Example
def my_function(**kwargs):
for key, value in kwargs.items():
print(key, value)
my_function(name="Alice", age=30, city="New York")
Output:
name Alice
age 30
city New York
In this example, the my_function() takes a variable number of keyword arguments using **kwargs. The for loop is used to iterate over the key-value pairs in the dictionary and print them out.
You can use both *args and **kwargs in the same function to accept both positional and keyword arguments:
Example using both *args and **kwargs
def my_function(*args, **kwargs):
for arg in args:
print(arg)
for key, value in kwargs.items():
print(key, value)
my_function("Hello", "world", name="Alice", age=30, city="New York")
Output:
Hello
world
name Alice
age 30
city New York
In this example, the my_function() accepts both positional arguments using *args and keyword arguments using **kwargs.
Therefore, *args and **kwargs are special syntax in Python that allow you to pass a variable number of arguments to a function. *args is used to pass a variable number of non-keyword (positional) arguments, while **kwargs is used to pass a variable number of keyword arguments. Using these special symbols makes your functions more flexible and versatile, and allows you to write code that can handle a wide range of input.