Integrate ChatGPT Into Your Website
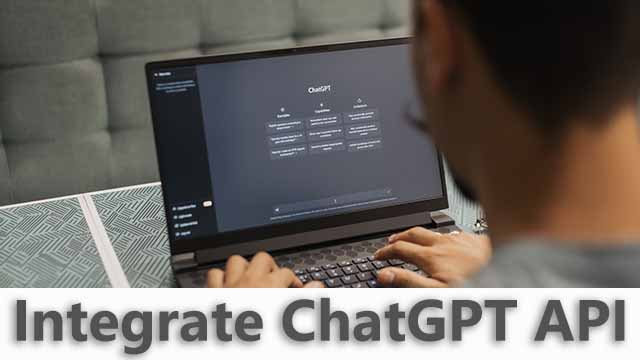
Integrating ChatGPT into your website can be a great way to provide an interactive experience for your users. In this blog post, we'll cover how to integrate ChatGPT into your website using the OpenAI API.
What is ChatGPT?
Before we dive into the technical details, let's briefly go over what ChatGPT is and how it works. ChatGPT is a language model trained by OpenAI that can understand natural language and generate human-like responses to text input. To use ChatGPT on your website, you'll need to connect to the OpenAI API, which allows you to send text input to the model and receive its responses.
Integrate ChatGPT Into Your Website
Now let's get into the steps you'll need to take to integrate ChatGPT into your website:
- Sign up for the OpenAI API: To use ChatGPT, you'll need to sign up for the OpenAI API. You can do this by visiting the OpenAI website and following the instructions to create an account. Once you've created an account, you'll need to provide some basic information about your project and agree to the API terms of service.
- Get an API key: After you've signed up for the OpenAI API, you'll need to get an API key to authenticate your requests. You can do this by visiting your OpenAI dashboard and clicking on the "API keys" tab. From there, you can create a new API key and copy it to your clipboard.
- Install the OpenAI Python client: To use the OpenAI API, you'll need to install the OpenAI Python client on your server. You can do this using pip, the Python package manager. Simply run the following command in your terminal:
pip install openai
- Write the integration code: Now it's time to write the code that will integrate ChatGPT into your website. Here's a simple example using Flask, a popular Python web framework:
-
from flask import Flask, request, jsonify import openai app = Flask(__name__) openai.api_key = 'YOUR_API_KEY_HERE' @app.route('/chat', methods=['POST']) def chat(): prompt = request.form['prompt'] response = openai.Completion.create(engine='davinci', prompt=prompt, max_tokens=1024) return jsonify({'response': response.choices[0].text}) if __name__ == '__main__': app.run()
This code defines a simple Flask app that listens for POST requests to the '/chat' endpoint. When it receives a request, it uses the OpenAI API to generate a response to the input prompt and returns the response as a JSON object.
Note that in this example, we're using the 'davinci' engine, which is the most powerful and expensive engine available on the OpenAI API. You can use a less powerful engine if you want to reduce costs.
-
- Deploy the integration code: Once you've written the integration code, you'll need to deploy it to a server that can handle incoming requests. There are many options for deploying Flask apps, including cloud services like Heroku or AWS, or self-hosting on your own server.
- Test the integration: Finally, you can test the integration by sending a POST request to your '/chat' endpoint with a prompt in the request body. You should receive a response from ChatGPT within a few seconds.
Congratulations, you've now integrated ChatGPT into your website! Of course, this is just a simple example, and you'll likely need to customize the integration code to fit your specific use case. But hopefully, this gives you a good starting point for integrating ChatGPT into your website.
Integrating ChatGPT into your website using PHP
Now, let's dive into the steps you'll need to take to integrate ChatGPT into your website using PHP code:
1. Sign up for the OpenAI API: To use ChatGPT, you'll need to sign up for the OpenAI API. You can do this by visiting the OpenAI website and following the instructions to create an account. Once you've created an account, you'll need to provide some basic information about your project and agree to the API terms of service.
2. Get an API key: After you've signed up for the OpenAI API, you'll need to get an API key to authenticate your requests. You can do this by visiting your OpenAI dashboard and clicking on the "API keys" tab. From there, you can create a new API key and copy it to your clipboard.
Install the OpenAI PHP client: To use the OpenAI API, you'll need to install the OpenAI PHP client on your server. You can do this using Composer, the PHP package manager. Simply add the following line to your composer.json file:
"require": {
"openai/openai": "^0.5.2"
}
Then run composer install in your terminal to install the package.
4. Write the integration code: Now it's time to write the code that will integrate ChatGPT into your website. Here's a simple example using PHP:
<?php
require __DIR__ . '/vendor/autoload.php';
use OpenAI\Api;
use OpenAI\Exception;
$api = new Api('YOUR_API_KEY_HERE');
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$prompt = $_POST['prompt'];
try {
$response = $api->completions([
'engine' => 'davinci',
'prompt' => $prompt,
'max_tokens' => 1024,
]);
$text = $response['choices'][0]['text'];
} catch (Exception $e) {
$text = $e->getMessage();
}
header('Content-Type: application/json');
echo json_encode(['response' => $text]);
exit;
}
?>
This code sets up the OpenAI API client, listens for POST requests to the page, and generates a response to the input prompt using the OpenAI API. It then returns the response as a JSON object.
Note that in this example, we're using the 'davinci' engine, which is the most powerful and expensive engine available on the OpenAI API. You can use a less powerful engine if you want to reduce costs.
5. Deploy the integration code: Once you've written the integration code, you'll need to deploy it to a server that can handle incoming requests. You can deploy this PHP code using a web server like Apache or Nginx.
6. Test the integration: Finally, you can test the integration by sending a POST request to your page with a prompt in the request body.
Here is an example of how to test the ChatGPT API integration using PHP. Here's an example code snippet:
// Set the API endpoint URL
$url = 'https://api.openai.com/v1/engine/davinci-codex/completions';
// Set the prompt for the API request
$data = array(
'prompt' => 'What is the meaning of life?',
'temperature' => 0.7,
'max_tokens' => 50
);
// Set the API headers
$headers = array(
'Content-Type: application/json',
'Authorization: Bearer YOUR_API_KEY'
);
// Send the API request using cURL
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec($curl);
// Print the API response
echo $response;
In the above code, replace YOUR_API_KEY with your actual API key. The prompt parameter is set to "What is the meaning of life?" for this example, but you can change this to any prompt you'd like to test with. The temperature and max_tokens parameters control the creativity and length of the response, respectively.
After running this code, you should receive a response from the ChatGPT API in JSON format, containing the generated text in the choices field. You can parse this response and use the generated text in your application as needed.
It's important to note that the ChatGPT API has rate limits in place, so be sure to check the API documentation for details on these limits and how to handle them in your application.
How much it cost to use ChatGPT API for my website?
The cost of using the ChatGPT API for your website depends on several factors, such as the number of requests you make, the size and complexity of the responses you receive, and the engine you use.
OpenAI offers several different pricing plans for its API, ranging from a free plan to custom enterprise plans. Here's a breakdown of the pricing for the different plans:
- Free plan: This plan includes 5,000 API requests per month for free. The requests are limited to the "davinci" engine and the response size is limited to 2048 tokens.
- Developer plan: This plan starts at $100 per month and includes 400,000 tokens per month. The developer plan includes access to all the engines, but there is a limit on the number of requests per minute.
- Team plan: This plan starts at $400 per month and includes 1.6 million tokens per month. The team plan includes higher rate limits than the developer plan and access to additional features like priority support.
- Business plan: This plan is designed for larger organizations and starts at $4,000 per month. It includes 16 million tokens per month and access to custom models, among other features.
- Enterprise plan: This plan is tailored to the specific needs of large enterprises and includes custom pricing and support.
It's important to note that these prices are subject to change, and you should check the OpenAI website for the most up-to-date pricing information.
Additionally, depending on your usage, there may be additional charges for exceeding your plan's token limits or for using custom models.
How to Integrate ChatGPT API into your Django application?
Integrating ChatGPT API into your Django application can be done using the requests library, which is a popular Python library for making HTTP requests. In this guide, we'll cover the steps you'll need to take to integrate ChatGPT into your Django application using Python and the requests library.
1. Sign up for the OpenAI API: The first step is to sign up for the OpenAI API if you haven't already done so. You can do this by visiting the OpenAI website and following the instructions to create an account.
2. Get an API key: After you've signed up for the OpenAI API, you'll need to get an API key to authenticate your requests. You can do this by visiting your OpenAI dashboard and clicking on the "API keys" tab. From there, you can create a new API key and copy it to your clipboard.
3. Install the requests library: You'll need to install the requests library to make HTTP requests to the OpenAI API. You can do this using pip by running the following command in your terminal:
pip install requests
4. Create a Django view: In your Django application, create a view that will handle incoming requests to generate responses from ChatGPT. Here's an example of a simple view that generates a response using the OpenAI API:
import json
import requests
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
@csrf_exempt
def generate_response(request):
if request.method == 'POST':
prompt = request.POST.get('prompt', '')
url = 'https://api.openai.com/v1/engines/davinci-codex/completions'
headers = {
'Content-Type': 'application/json',
'Authorization': f'Bearer {YOUR_API_KEY_HERE}',
}
data = {
'prompt': prompt,
'max_tokens': 1024,
'n': 1,
'stop': '\n',
}
response = requests.post(url, headers=headers, data=json.dumps(data))
response_json = response.json()
text = response_json['choices'][0]['text']
return JsonResponse({'response': text})
else:
return JsonResponse({})
5. Configure your Django URLs: Once you've created your view, you'll need to configure your Django URLs to route incoming requests to the view. Here's an example of how to configure your URLs:
from django.urls import path
from .views import generate_response
urlpatterns = [
path('generate_response/', generate_response, name='generate_response'),
]
6. Test the integration: Finally, you can test the integration by sending a POST request to your Django application with a prompt in the request body. You should receive a response from the OpenAI API containing a generated response.
That's it! With these steps, you should be able to integrate ChatGPT API into your Django application and generate human-like responses to text input.
What else you can ask more about ChatGPT API?
Here are some additional topics related to the ChatGPT API that you could ask about:
- How to use the different GPT-3 models: The ChatGPT API provides access to several GPT-3 models, each with different capabilities and use cases. You could ask about how to choose the right model for your specific application or how to use the different models to achieve different results.
- How to fine-tune the GPT-3 models: Fine-tuning is the process of training a pre-trained model on a specific dataset to make it more accurate for a specific task. You could ask about how to fine-tune the GPT-3 models for your specific use case, or how to train your own custom models using the OpenAI API.
- How to use the API with other programming languages: While we covered integrating ChatGPT into a Django application using Python, you could also ask about how to integrate the API into applications built with other programming languages, such as JavaScript, Java, or Ruby.
- How to handle API rate limits: The OpenAI API has rate limits in place to prevent abuse and ensure fair usage. You could ask about how to handle rate limits in your application and what strategies you can use to avoid hitting the limits.
- How to ensure the safety and ethical use of the API: The ChatGPT API has the potential to generate realistic, human-like responses to text input, which can be used for both good and bad purposes. You could ask about how to ensure the safety and ethical use of the API in your application and what measures you can take to prevent malicious use.
ChatGPT is a powerful API that provides developers with the ability to generate human-like responses to text input. The API is built on top of the GPT-3 language model, which is one of the most advanced natural language processing models available today.
Using ChatGPT, developers can create chatbots, virtual assistants, and other conversational interfaces that can interact with users in a natural and intuitive way. The API is easy to use and can be integrated into a wide range of applications, including websites, mobile apps, and messaging platforms.
However, as with any AI-based technology, there are also ethical and safety concerns to consider when using ChatGPT. It's important for developers to be mindful of the potential risks and to design their applications in a way that prioritizes user privacy and safety.
Overall, ChatGPT is an exciting technology that has the potential to revolutionize the way we interact with machines. With careful planning and responsible use, it can be a valuable tool for creating more engaging and intuitive user experiences.
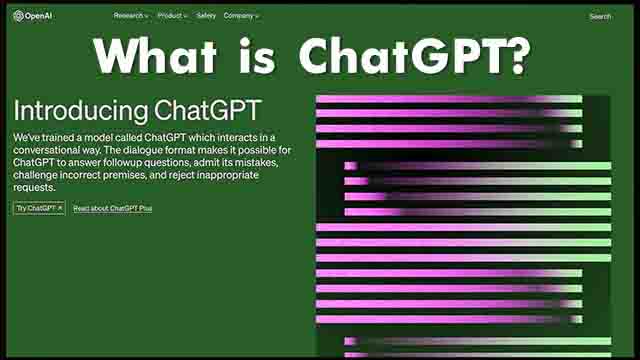
ChatGPT is a cutting-edge AI-powered chatbot that utilizes natural language processing to interact with users in a human-like manner. It can answer... read more