Datetime in Python
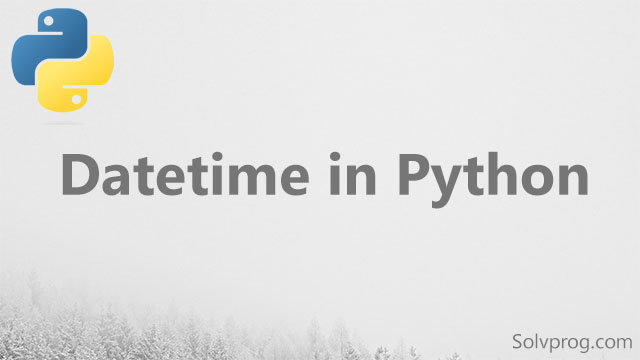
Python is a widely used programming language that has many useful built-in modules. One of these modules is the datetime module, which provides classes for working with dates and times. The datetime module is a powerful tool for handling time-related data and provides a lot of functionality.
In this blog post, we will explore the datetime module and learn how to work with dates and times in Python. We will cover topics such as creating datetime objects, formatting dates and times, and performing date and time arithmetic. We will also provide plenty of examples to help illustrate each concept.
Example
First Importing the datetime module and then displaying the current date:
import datetime
x = datetime.datetime.now()
print(x)
Creating Datetime Objects
The datetime module provides several classes for working with dates and times. The most commonly used classes are date, time, and datetime. Here is a brief overview of each class:
- date: Represents a date (year, month, and day).
- time: Represents a time of day (hour, minute, second, and microsecond).
- datetime: Represents a date and time (year, month, day, hour, minute, second, and microsecond).
To create a datetime object, you can use the datetime() constructor, which takes arguments for the year, month, day, hour, minute, second, and microsecond. Here is an example:
from datetime import datetime
dt = datetime(2023, 4, 18, 9, 30, 0)
print(dt)
Output:
2023-04-18 09:30:00
You can also create a datetime object from a string using the strptime() method. This method takes two arguments: a string representing the date and time and a string representing the format of the date and time. Here is an example:
from datetime import datetime
dt_str = '2023-04-18 09:30:00'
dt = datetime.strptime(dt_str, '%Y-%m-%d %H:%M:%S')
print(dt)
Output:
2023-04-18 09:30:00
In this example, we use the format string %Y-%m-%d %H:%M:%S to indicate the format of the date and time string.
Formatting Dates and Times
The strftime() method can be used to format dates and times. This method takes a format string as its argument and returns a string representing the date and time in the specified format. Here is an example:
from datetime import datetime
dt = datetime(2023, 4, 18, 9, 30, 0)
dt_str = dt.strftime('%Y-%m-%d %H:%M:%S')
print(dt_str)
Output:
2023-04-18 09:30:00
In this example, we use the format string %Y-%m-%d %H:%M:%S to format the datetime object as a string.
Performing Date and Time Arithmetic
The datetime module provides several methods for performing date and time arithmetic. These methods include timedelta(), replace(), weekday(), and more.
The timedelta() method can be used to add or subtract a duration from a datetime object. Here is an example:
from datetime import datetime, timedelta
dt = datetime(2023, 4, 18, 9, 30, 0)
delta = timedelta(days=7)
new_dt = dt + delta
print(new_dt)
Output:
2023-04-25 09:30:00
In this example, we use the timedelta() method to add 7 days to the datetime object dt.
The replace() method can be used to change specific attributes of a datetime object. Here is an example:
from datetime import datetime
dt = datetime(2023, 4, 18, 9, 30, 0)
new_dt = dt.replace(year=2024)
print(new_dt)
Output:
2024-04-18 09:30:00
In this example, we use the replace() method to change the year attribute of the datetime object dt to 2024.
The weekday() method can be used to get the day of the week for a given date. Here is an example:
from datetime import datetime
dt = datetime(2023, 4, 18, 9, 30, 0)
weekday = dt.weekday()
print(weekday)
Output:
1
In this example, we use the weekday() method to get the day of the week for the datetime object dt. Note that the method returns an integer, with Monday being 0 and Sunday being 6.
Conclusion
The datetime module provides a lot of functionality for working with dates and times in Python. We have covered some of the most important concepts in this blog post, including creating datetime objects, formatting dates and times, and performing date and time arithmetic. By using these concepts, you can easily manipulate and work with time-related data in your Python programs.