Python JSON
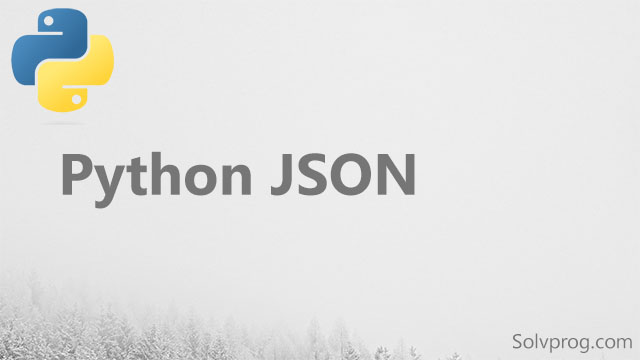
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. In Python, the json module provides functionality for working with JSON data. This blog post will cover the following topics:
- JSON basics
- Parsing JSON data with Python
- Generating JSON data with Python
- Working with complex JSON data structures
JSON Basics
JSON data is represented as key-value pairs, similar to Python dictionaries. Here is an example of a JSON object:
{
"name": "John",
"age": 30,
"city": "New York"
}
In this example, the JSON object has three key-value pairs: name, age, and city. The keys are always strings, and the values can be strings, numbers, arrays, or other JSON objects.
JSON arrays are represented as square brackets containing comma-separated values. Here is an example of a JSON array:
[
"apple",
"banana",
"cherry"
]
In this example, the JSON array has three values: "apple", "banana", and "cherry".
Parsing JSON Data with Python
To parse JSON data with Python, we can use the json.loads() method, which takes a JSON string as input and returns a Python object:
import json
# JSON string
json_str = '{"name": "John", "age": 30, "city": "New York"}'
# Parse JSON data
data = json.loads(json_str)
# Print data
print(data)
Output:
{'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we use the json.loads() method to parse a JSON string and convert it into a Python object. We then print the resulting object, which is a Python dictionary.
To parse JSON data from a file, we can use the json.load() method, which takes a file object as input and returns a Python object:
import json
# Open JSON file
with open('data.json', 'r') as f:
# Parse JSON data
data = json.load(f)
# Print data
print(data)
In this example, we use the open() function to open a JSON file and read its contents. We then use the json.load() method to parse the JSON data and convert it into a Python object. Finally, we print the resulting object.
Generating JSON Data with Python
To generate JSON data with Python, we can use the json.dumps() method, which takes a Python object as input and returns a JSON string:
import json
# Python object
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# Generate JSON data
json_data = json.dumps(data)
# Print JSON data
print(json_data)
Output:
{"name": "John", "age": 30, "city": "New York"}
In this example, we use the json.dumps() method to generate JSON data from a Python dictionary. We then print the resulting JSON string.
To generate JSON data and write it to a file, we can use the json.dump() method, which takes a Python object and a file object as input:
import json
# Python object
data = {
'name': 'John',
'age': 30,
'city': 'New York'
}
# Write JSON data to file
with open('data.json', 'w') as f:
json.dump(data, f)
In this example, we define a Python object called data that represents a person's name, age, and city. We then use the open() function to create a new file called data.json in write mode, and we use the json.dump() method to write the JSON data to the file.
It simply writes the JSON data to the file data.json.
To confirm that the data has been written to the file, you can open the file in a text editor or use the following code to read the contents of the file:
import json
# Read JSON data from file
with open('data.json', 'r') as f:
data = json.load(f)
# Print Python object
print(data)
This will read the data from the data.json file and print it to the console. The output will be:
{'name': 'John', 'age': 30, 'city': 'New York'}
Working with Complex JSON Data Structures
JSON data can also include complex data structures, such as nested objects and arrays. Here is an example of a JSON object that includes both:
{
"name": "John",
"age": 30,
"city": "New York",
"pets": [
{
"name": "Fido",
"type": "dog"
},
{
"name": "Fluffy",
"type": "cat"
}
]
}
In this example, the JSON object includes a nested array of objects called pets. Each object in the array represents a pet with a name and a type.
To access values in a nested object or array, we can use the [] operator to chain multiple keys or indexes together:
import json
# JSON string
json_str = '''
{
"name": "John",
"age": 30,
"city": "New York",
"pets": [
{
"name": "Fido",
"type": "dog"
},
{
"name": "Fluffy",
"type": "cat"
}
]
}
'''
# Parse JSON data
data = json.loads(json_str)
# Access nested values
print(data['name'])
print(data['pets'][0]['name'])
Output:
John
Fido
In this example, we use the [] operator to access the name value in the top-level object and the name value in the first object in the pets array.
We can also modify values in a JSON object by assigning a new value to a key:
import json
# JSON string
json_str = '''
{
"name": "John",
"age": 30,
"city": "New York",
"pets": [
{
"name": "Fido",
"type": "dog"
},
{
"name": "Fluffy",
"type": "cat"
}
]
}
'''
# Parse JSON data
data = json.loads(json_str)
# Modify value
data['age'] = 40
# Generate JSON data
json_data = json.dumps(data)
# Print JSON data
print(json_data)
Output:
{"name": "John", "age": 40, "city": "New York", "pets": [{"name": "Fido", "type": "dog"}, {"name": "Fluffy", "type": "cat"}]}
In this example, we use the [] operator to modify the value of the age key in the top-level object. We then use the json.dumps() method to generate new JSON data from the modified Python object.
Conclusion
In this blog post, we covered the basics of working with JSON data in Python, including parsing JSON data with json.loads() and json.load(), generating JSON data with json.dumps() and json.dump(), and working with complex JSON data structures. JSON is a powerful and flexible data format that is widely used in web development and data exchange, and Python's json module provides a simple and convenient way to work with JSON data in your applications.