Python User Input
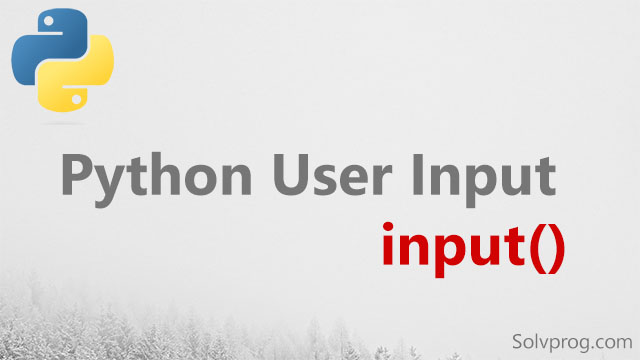
In Python, we can use the input() function to get user input from the console. The input() function takes a single argument, which is a prompt message to display to the user. The user can then type in their response and press Enter.
Python Input() Function
In Python, we can use the input() function to take user input from the console. The input() function takes a single argument, which is a prompt message to display to the user. The user's input is returned as a string.
Here is an example of using the input() function to ask the user for their name:
name = input("What is your name? ")
print("Hello, " + name + "!")
In this code, we use the input() function to ask the user for their name. The prompt message "What is your name?" is displayed in the console. The user's input is then stored in the variable 'name'. Finally, we use the print() function to display a message that greets the user by name.
Here are some more examples of using the input() function:
# Example 1: Asking for a number
number = input("Enter a number: ")
print("You entered " + number)
# Example 2: Asking for a yes/no answer
answer = input("Do you like Python? (y/n) ")
if answer.lower() == "y":
print("Great, me too!")
else:
print("Oh well, to each their own.")
# Example 3: Asking for multiple inputs
name = input("What is your name? ")
age = input("How old are you? ")
print("Your name is " + name + " and you are " + age + " years old.")
In example 1, we ask the user to enter a number. The user's input is stored in the variable number, and we use the print() function to display a message that includes the user's input.
In example 2, we ask the user if they like Python using a yes/no question. We convert the user's input to lowercase using the lower() method, and then use an if statement to check if the user answered "y". If they did, we print a message indicating that we also like Python. If they answered anything else, we print a message indicating that we respect their opinion.
In example 3, we ask the user for their name and age using two separate input() calls. We store the user's input in the variables name and age, and then use the print() function to display a message that includes the user's name and age.
We can also use the input() function in combination with control structures like if statements and loops to create interactive programs that respond to user input. Here is an example of using the input() function in an if statement:
answer = input("Do you like Python? (yes/no) ")
if answer == "yes":
print("Great, me too!")
else:
print("Oh well, to each their own.")
In this code, we ask the user if they like Python using the input() function. We then use an if statement to check if the user answered "yes". If they did, we print a message indicating that we also like Python. If they answered anything else, we print a message indicating that we respect their opinion.
Overall, the input() function is a powerful tool in Python that allows us to create interactive programs that respond to user input. By combining the input() function with control structures and data types, we can create programs that are both flexible and user-friendly.