PHP Sorting Arrays: Mastering Array Sorting in PHP
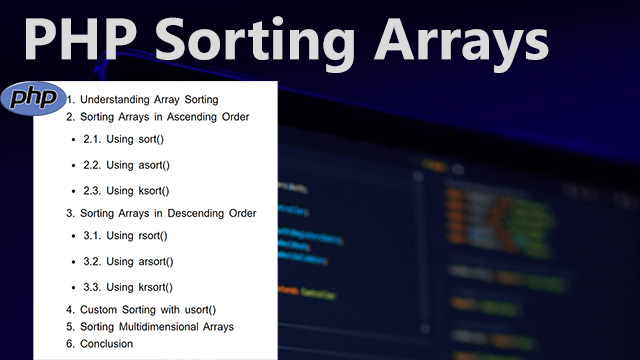
Sorting arrays is a fundamental operation in programming, allowing you to arrange data in a specific order for easier processing and retrieval. In PHP, there are various techniques and functions available to sort arrays efficiently. In this article, we will explore the different methods and dive into examples to showcase their usage. By the end, you'll have a solid understanding of PHP array sorting and be equipped to handle array data like a pro.
Table of Contents:
- Understanding Array Sorting
- Sorting Arrays in Ascending Order
- 2.1. Using sort()
- 2.2. Using asort()
- 2.3. Using ksort()
- Sorting Arrays in Descending Order
- 3.1. Using rsort()
- 3.2. Using arsort()
- 3.3. Using krsort()
- Custom Sorting with usort()
- Sorting Multidimensional Arrays
- Conclusion
1: Understanding Array Sorting:
Before we dive into the various sorting methods, let's establish a basic understanding of array sorting in PHP. Array sorting refers to the process of rearranging the elements of an array based on specific criteria, such as numerical or alphabetical order. PHP provides several built-in functions to facilitate this process, making it efficient and hassle-free.
2: Sorting Arrays in Ascending Order:
In this section, we will explore three common methods for sorting arrays in ascending order: sort(), asort(), and ksort(). These functions sort arrays based on their values, preserving the association between keys and values.
2.1. Using sort():
The sort() function arranges the elements of an array in ascending order based on their values. It modifies the original array directly. Here's an example to illustrate its usage:
$numbers = [7, 2, 9, 4, 1];
sort($numbers);
print_r($numbers);
Output:
Array
(
[0] => 1
[1] => 2
[2] => 4
[3] => 7
[4] => 9
)
2.2. Using asort():
The asort() function is similar to sort(), but it preserves the key-value association. It sorts the array based on values while keeping the original keys intact. Let's see it in action:
$students = [
'John' => 25,
'Alice' => 20,
'Bob' => 23,
'Eve' => 21
];
asort($students);
print_r($students);
Output:
Array
(
[Alice] => 20
[Eve] => 21
[Bob] => 23
[John] => 25
)
2.3. Using ksort():
The ksort() function sorts an array based on its keys while maintaining the corresponding values. It rearranges the elements in ascending order based on keys. Consider the following example:
$fruits = [
'banana' => 2,
'apple' => 3,
'orange' => 1,
'grape' => 4
];
ksort($fruits);
print_r($fruits);
Output:
Array
(
[apple] => 3
[banana] => 2
[grape] => 4
[orange] => 1
)
3: Sorting Arrays in Descending Order:
While we've explored sorting arrays in ascending order, PHP also provides functions to sort arrays in descending order. Let's delve into the rsort(), arsort(), and krsort() functions.
3.1. Using rsort():
The rsort() function arranges the elements of an array in descending order based on their values. It modifies the original array directly. Let's examine an example:
$numbers = [7, 2, 9, 4, 1];
rsort($numbers);
print_r($numbers);
Output:
Array
(
[0] => 9
[1] => 7
[2] => 4
[3] => 2
[4] => 1
)
3.2. Using arsort():
The arsort() function is similar to rsort(), but it preserves the key-value association. It sorts the array based on values while keeping the original keys intact. Let's see it in action:
$students = [
'John' => 25,
'Alice' => 20,
'Bob' => 23,
'Eve' => 21
];
arsort($students);
print_r($students);
Output:
Array
(
[John] => 25
[Bob] => 23
[Eve] => 21
[Alice] => 20
)
3.3. Using krsort():
The krsort() function sorts an array based on its keys in descending order while maintaining the corresponding values. It rearranges the elements based on keys from highest to lowest. Consider the following example:
$fruits = [
'banana' => 2,
'apple' => 3,
'orange' => 1,
'grape' => 4
];
krsort($fruits);
print_r($fruits);
Output:
Array
(
[orange] => 1
[grape] => 4
[banana] => 2
[apple] => 3
)
4: Custom Sorting with usort():
PHP also allows you to perform custom sorting using the usort() function. This function enables you to define your own comparison logic to sort the array according to specific criteria. Let's look at an example:
$fruits = ['apple', 'banana', 'grape', 'orange'];
usort($fruits, function ($a, $b) {
return strlen($a) - strlen($b);
});
print_r($fruits);
Output:
Array
(
[0] => apple
[1] => grape
[2] => banana
[3] => orange
)
5: Sorting Multidimensional Arrays:
Sorting multidimensional arrays requires special attention. In such cases, you can use the array_multisort() function to sort multiple arrays or columns within an array simultaneously. Let's illustrate this with an example:
$students = [
['name' => 'John', 'age' => 25],
['name' => 'Alice', 'age' => 20],
['name' => 'Bob', 'age' => 23],
['name' => 'Eve', 'age' => 21]
];
// Sort based on age in ascending order
array_multisort(array_column($students, 'age'), $students);
print_r($students);
Output:
Array
(
[0] => Array
(
[name] => Alice
[age] => 20
)
[1] => Array
(
[name] => Eve
[age] => 21
)
[2] => Array
(
[name] => Bob
[age] => 23
)
[3] => Array
(
[name] => John
[age] => 25
)
)
6: Summary
We have covered a wide range of methods and PHP functions for sorting arrays in this extensive article. You now have a thorough grasp of PHP array sorting, including how to sort arrays in ascending or descending order, conduct custom sorting, and work with multidimensional arrays. You can easily arrange and modify your array data by using these methods. So go ahead and use PHP array sorting to its full potential in your applications!
Keep in mind to consult the official PHP documentation for more details on array sorting and to explore the other possible sorting methods and options.
Coding is fun!