How to Create an App in Django Project?
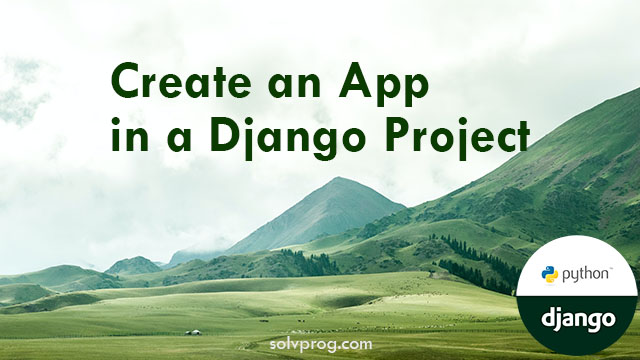
In Django, a project is like a complete website, and an application is a specific part or feature of that website. A project represents the overall configuration and settings for a web application, while an application is a modular component that contains related functionality, such as handling user authentication, managing blog posts, or serving static content.
A Django project is a collection of settings, database configurations, and URL patterns that make up the entire web application. It acts as the container for one or more applications.
On the other hand, a Django application is a self-contained unit that performs a specific task within the project. It consists of models, views, templates, and URL patterns. Each application represents a particular feature or functionality that can be reused across different projects.
To create a complete website, you can have multiple applications working together within a single project.
For example, a blogging website may have separate applications for user authentication, blog posts, comments, and categories.
By dividing your project into multiple applications, you can achieve better organization, reusability, and maintainability of your codebase. It allows you to focus on building specific features in isolation and then integrating them into the overall project.
Overall, a Django project provides the structure and configuration, while applications implement specific functionalities, resulting in a well-organized and scalable web application.
What is an App?
An app is a small, specific part of a web application that serves a particular purpose. It can be anything from a homepage to a contact form or a user database.
In this tutorial, we'll create an app that helps us manage members in a database, allowing us to list and register them.
But before that, let's start with a simple Django app that just displays the static message "Welcome, Hello World!" on the web page. This will give us a basic understanding of how apps work in Django.
Create an App
Before, you create an app inside a django project, choose a name of your app. I will name it as "todoapp". From this title we learn that the name should match the functionality of an app, so it's fast and easy for you to work on your project.
1. Open your terminal or command prompt.
2. Navigate to the location where you have a project folder "myproject". It needs to be inside a project folder.
For example, if you have a folder named "myproject," use the "cd" command to change into that folder:
cd myproject
Now we will create an app using the "startapp" command followed by the app name:
python manage.py startapp todoapp
That’ll create a directory named todoapp, which is going to look like this:
todoapp/
__init__.py
admin.py
apps.py
migrations/
__init__.py
models.py
tests.py
views.py
After creating the app and defining the models, views, and templates, you need to register the app in your Django project with settings.py and specify its URL patterns in urls.py.
Register the todoapp App in "settings.py":
Open the "settings.py" file in your Django project directory (not the app directory). Look for the INSTALLED_APPS setting and add your app's name to the list of installed apps:
INSTALLED_APPS = [
# Other installed apps...
'todoapp', # Add your app's name here
]
Create URLs for the App:
In the app directory ("todoapp" directory), create a file named "urls.py". if it doesn't already exist. In this file, define the URL patterns specific to your app. For example:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'), # Replace 'index' with your view function name
# Add more URL patterns for other views as needed
]
- We import Django's path function and your app's views module.
- The URL pattern is defined using the path function.
- The first argument of path is the URL pattern (e.g., '/' for the homepage).
- The second argument is the view function that will handle the request (e.g., index).
- You can replace 'index' with the name of your actual view function.
- An optional name can be provided to the pattern for easier reference in templates.
By setting up the URL pattern, Django knows which view function to call when a user visits the specified URL. This allows the app to respond to user requests and display the appropriate content on the web page.
Include App URLs in Project URLs:
In the main "urls.py" file of your Django project (usually located in the project's directory "myproject"), include your app's URLs. This is done using the include function from django.urls:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('todoapp/', include('todoapp.urls')), # Replace 'todoapp' with your app's name
]
By including your app's URLs in the main urlpatterns, you make sure that when a user visits the "/todoapp/" URL (replace with your desired URL), Django will route the request to the views defined in your app.
That's it! Now, your app is registered in your Django project, and you've specified the URL patterns for the app. You can access your app by navigating to the specified URL in your web browser. Don't forget to replace 'todoapp' with your actual app's name in the above code snippets.