How to create a responsive table with HTML and CSS?
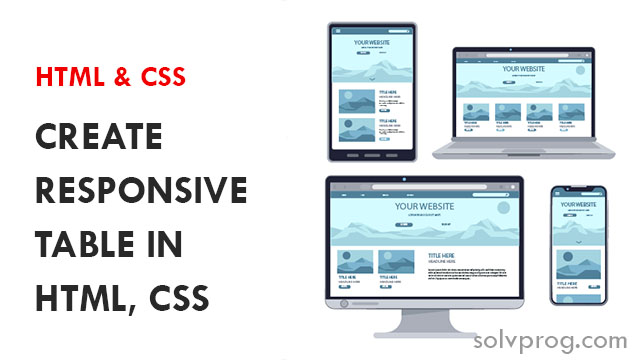
Responsive web design is about making your website look good on all devices by adjusting and rearranging its elements to fit various screen sizes, ensuring a better user experience. In this post, we will create a responsive table using HTML and CSS.
We'll focus on making tables responsive using CSS. When dealing with tables that have a lot of data, making them responsive is essential for readability. To achieve this, we can use a CSS property called "overflow-x."
The "overflow-x" property allows us to control how the table behaves when it overflows its container horizontally (left and right). We can use it to add a scroll bar to the table or slice the data, ensuring that users can read the table content comfortably without stretching the screen.
By making the table responsive, we improve user experience, making it easier for people to read and interact with the data, no matter the device they're using. This contributes to a more enjoyable and user-friendly website.
Create a responsive table
Step 1: Add a class to the table
Inside the opening <table> tag, add the class "table" and "table-responsive" like this:
<table class="table table-responsive">
<!-- Your table content goes here -->
</table>
Step 2: Wrap the table inside a container
Next, wrap the entire table inside a <div> element and apply the CSS property "overflow-x" with the value "auto" to this container. This will enable horizontal scrolling for the table when needed:
<div style="overflow-x: auto;">
<table class="table table-responsive">
<!-- Your table content goes here -->
</table>
</div>
Using these simple methods, you will be able to generate a responsive table, making its responsive size and allowing for horizontal scrolling as needed. Several platforms, such as desktops, tablets, and phones, may easily access and interact with the table information, resulting in a better overall user experience.
Example
In this code snippet, we have a table with various columns and rows. To make the table responsive and enhance the user experience on different devices, we apply the CSS style "overflow-x:auto." This cleverly adjusts the table to fit the screen size, ensuring a seamless user experience.
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
table {
width: 100%;
border: 1px solid blue;
}
th, td {
text-align: left;
padding: 8px;
}
</style>
</head>
<body>
<h2>CSS Responsive Table</h2>
<div style="overflow-x:auto;">
<table>
<tr>
<th>Product</th>
<th>Category</th>
<th>Price</th>
<th>Stock</th>
<th>Supplier</th>
<th>Rating</th>
<th>Reviews</th>
<th>Discount</th>
<th>Featured</th>
<th>New Arrivals</th>
<th>Colors</th>
<th>Sizes</th>
</tr>
<tr>
<td>Widget A</td>
<td>Electronics</td>
<td>150</td>
<td>25</td>
<td>ABC Electronics</td>
<td>4.5</td>
<td>100</td>
<td>10%</td>
<td>Yes</td>
<td>No</td>
<td>Black, Silver</td>
<td>S, M, L</td>
</tr>
<tr>
<td>Gadget B</td>
<td>Gadgets</td>
<td>250</td>
<td>10</td>
<td>XYZ Gadgets</td>
<td>4.8</td>
<td>200</td>
<td>15%</td>
<td>No</td>
<td>Yes</td>
<td>Blue, Red</td>
<td>One Size</td>
</tr>
<tr>
<td>Apparel C</td>
<td>Fashion</td>
<td>80</td>
<td>50</td>
<td>FashionHub</td>
<td>4.2</td>
<td>70</td>
<td>20%</td>
<td>Yes</td>
<td>No</td>
<td>White, Blue, Pink</td>
<td>S, M, L, XL</td>
</tr>
</table>
</div>
</body>
</html>
Example
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
table {
border-collapse: collapse;
border-spacing: 0;
width: 100%;
border: 1px solid #ddd;
}
th, td {
text-align: left;
padding: 8px;
}
tr:nth-child(even){background-color: #f2f2f2}
</style>
</head>
<body>
<h2>Responsive Table Example</h2>
<p>This is a responsive table that adjusts its size to fit different devices. If the table is too wide, it will show a horizontal scroll bar for easy navigation.</p>
<p>Try resizing your browser window to see how the table adapts. You can also experiment by removing the surrounding div element to observe the changes in the table layout.</p>
<div style="overflow-x:auto;">
<table>
<tr>
<th>Subject</th>
<th>Student A</th>
<th>Student B</th>
<th>Student C</th>
<th>Student D</th>
<th>Student E</th>
<th>Student F</th>
<th>Student G</th>
<th>Student H</th>
<th>Student I</th>
<th>Student J</th>
<th>Total Marks</th>
</tr>
<tr>
<td>Maths</td>
<td>95</td>
<td>88</td>
<td>90</td>
<td>92</td>
<td>85</td>
<td>94</td>
<td>87</td>
<td>91</td>
<td>89</td>
<td>90</td>
<td>901</td>
</tr>
<tr>
<td>Science</td>
<td>88</td>
<td>92</td>
<td>86</td>
<td>94</td>
<td>90</td>
<td>92</td>
<td>87</td>
<td>93</td>
<td>91</td>
<td>89</td>
<td>902</td>
</tr>
<tr>
<td>History</td>
<td>85</td>
<td>90</td>
<td>91</td>
<td>88</td>
<td>86</td>
<td>94</td>
<td>89</td>
<td>92</td>
<td>90</td>
<td>88</td>
<td>894</td>
</tr>
</table>
</div>
</body>
</html>
To create a responsive table, simply wrap the <table> inside a container element (like a <div>) and apply the style "overflow-x:auto" to the container. This is going to add a horizontal scroll bar when the table becomes too wide, making it easy to view the content on different devices. The table will adjust and fit perfectly, ensuring a better user experience.
In conclusion, a responsive table is crucial for a better user experience on all devices. By using "overflow-x:auto" around the table, it adapts to different screen sizes, ensuring readability and usability. Responsive design is important for making websites accessible to a wider audience, regardless of the device they use.
Remember, a positive user experience is key to a successful website, so always prioritize responsiveness to create a user friendly browsing experience for all visitors.