PHP Variable $this keyword
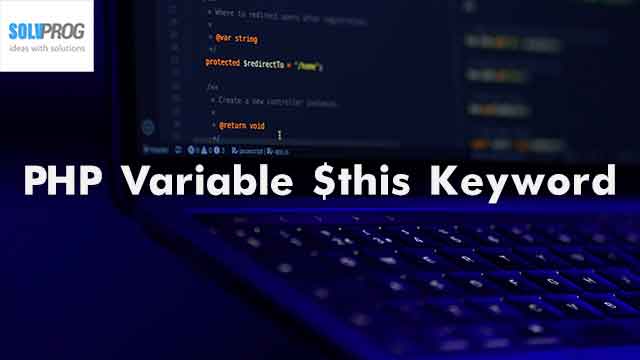
$this keyword in PHP is a reserved keyword that reference to the current object, it's most commonly used in object oriented code.
It is generally the object to which the method belongs, although it might be another object if called statically from the context of a secondary object. This keyword applies solely to internal methods.
Example 1: A simple program to show the use of $this in PHP.
Filename: php-variable-this-keyword.php
<?php
class Person {
public $name;
function __construct( $name ) {
$this->name = $name;
}
};
$myname = new Person('Sagar');
echo $myname->name;
?>
This stores the 'Sagar' string as a property of the object created.
Output:
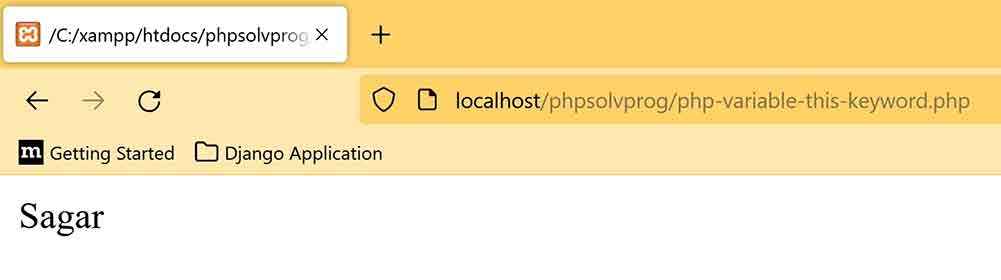
Example 1: Output $this keyword
Example 2.
Filename: php-variable-this-keyword.php
<?php
class Numbr{
public $n = 9;
public function display(){
return $this->n;
}
}
$displayNumbr = new Numbr();
echo $displayNumbr->display();
?>
Output:
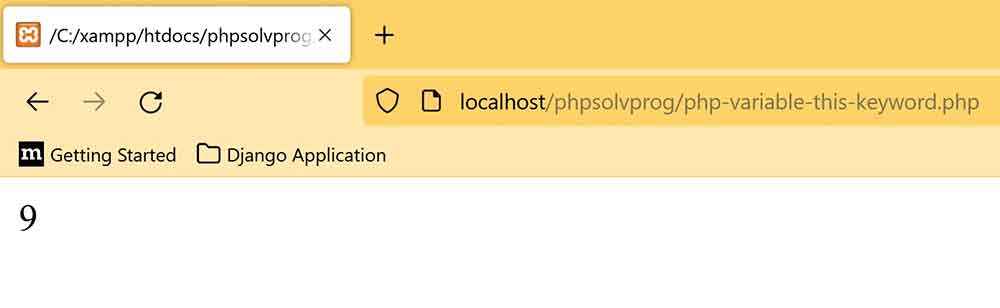
Example 2: Output $this keyword
Example 3.
Filename: php-variable-this-keyword.php
<?php
class Numbr {
public $n = 9;
public function change($val){
$this->n = $val;
}
public function display(){
return $this->n;
}
}
$displayNumbr = new Numbr();
print("value of before update: ");
echo $displayNumbr->display();
echo "<br/>";
$displayNumbr->change(7);
print("value of after update: ");
echo $displayNumbr->display();
?>
Output:
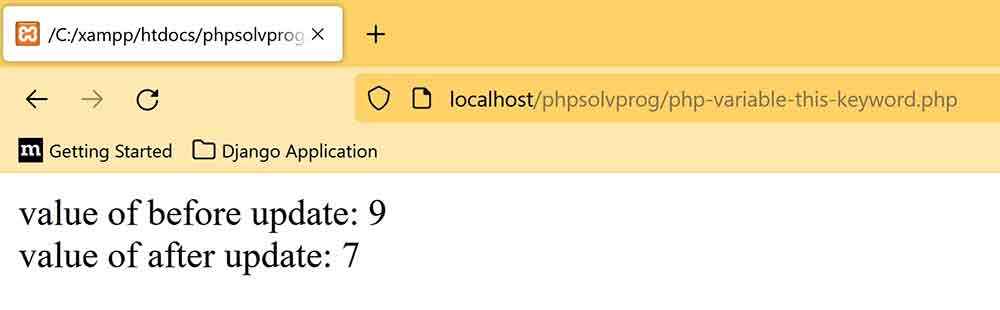
Example 3: Output $this keyword
What does the variable $this mean in PHP?
The pseudo-variable $this is available when a method is called from within an object context. $this is a reference to the calling object (usually the object to which the method belongs, but possibly another object, if the method is called statically from the context of a secondary object).
Lets see what happens if we won't use $this and try to have instance variables and constructor arguments with the same name with the following code snippet.
Example: using code without $this keyword
Filename: php-variable-this-keyword.php
<?php
class Student {
public $name;
function __construct( $name ) {
$name = $name;
}
};
$myname = new Student('Sagar');
echo $myname->name;
?>
It displays out on the screen nothing but
Example: using $this keyword in PHP given code
Filename: php-variable-this-keyword.php
<?php
class Student {
public $name;
function __construct( $name ) {
$this->name = $name; // Using 'this' to access the student's name
}
};
$myname = new Student('Sagar');
echo $myname->name;
?>
The result output is: 'Sagar'
PHP OOP $this Keyword
Filename: php-variable-this-keyword.php
<?php
class Phone {
public $color = 'white';
}
$blackPhone = new Phone();
?>
Where to use $this?
How can we change the value of $color? Take some time and think.
There are two ways.
Outside the class. We can directly change the value of a property from outside of the class.
$blackPhone -> color = 'black';
Inside the class. We can define a method in the class and call it to change the value of its own property.
public function changeColor() {
$this -> color = 'black';
}
$blackPhone -> changeColor();
In the second way, we use $this variable to access the current object. Here, the current object is the object saved in $blackPhone.
Complete Code Example:
Filename: php-variable-this-keyword.php
<?php
class Phone {
public $name;
public $color;
public function setList($name, $color) {
$this -> name = $name;
$this -> color = $color;
}
public function echoList() {
echo "The color of the {$this -> name} is {$this -> color}";
}
}
$blackPhone = new Phone();
$whitePhone = new Phone();
$blackPhone -> setList("iPhone", "black");
$whitePhone -> setList("Samsung", "white");
$blackPhone -> echoList();
echo '<br>';
$whitePhone -> echoList();
?>
Output:
The color of the iPhone is black
The color of the Samsung is white
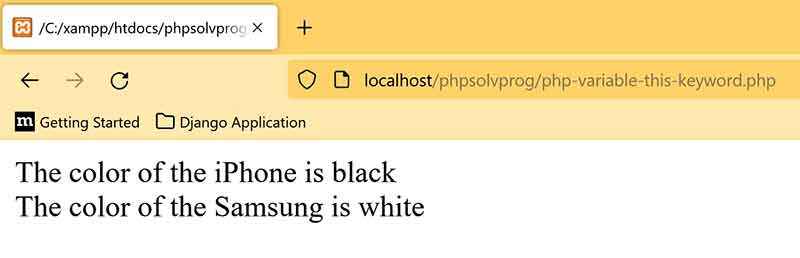
Complete Code: Output $this keyword
Conclusion
We could access the current object with $this keyword. But, we needed to run two steps of code to create a object ($blackPhone = new Phone()) and set its list ($blackPhone -> setList(...)). What if we could set the data when we create the object? We can run a constructor function when the object is created. As you can see in previous examples given.