PHP Variable Scope
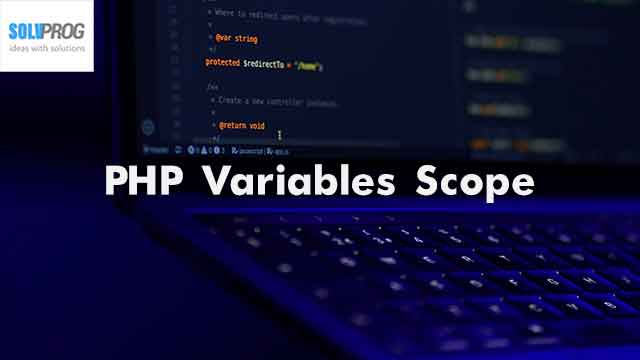
The scope of a variable is defined by a range or its boundary in a program within which it can be accessed from code. The scope of a variable is the part of the script where the variable can be accessed.
PHP has three types of variable scopes:
- Local variable
- Global variable
- Static variable
1. Local Variables Scope
Variables declared within a function are referred to as local variables for that function. These local variables have no scope outside of the function in which they are defined. Because these variables have limited scope, they cannot be accessed outside of the function.
A variable declared within a function has a LOCAL VARIABLE SCOPE and can only be accessed from within that function:
Filename: php-variable-scope.php
<?php
function Scope() {
$number = 5; // local scope
echo "<p>Variable number inside function is: $number</p>";
}
Scope();
// using variable number outside the function will generate an error
echo "<p>Variable number outside function is: $number</p>";
?>
Output:
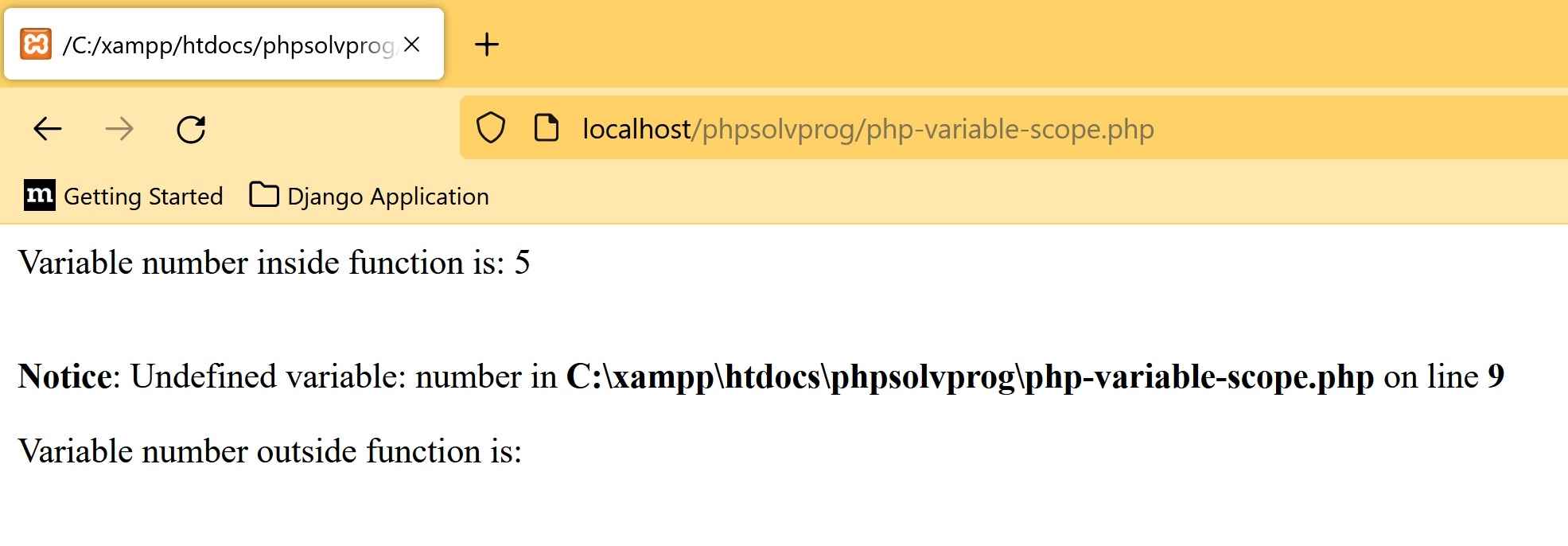
Output: Local variable scope
2. Global variable scope
Global variables are those that are declared outside of the function. These variables are accessible throughout the application. Use the GLOBAL keyword before the variable to access the global variable within a function. These variables, however, can be accessed or used straight outside of the function without the usage of a keyword. As a result, no keyword is required to access a global variable outside of the function.
A variable declared outside a function has a GLOBAL VARIABLE SCOPE and can only be accessed from outside a function:
Filename: php-variable-scope.php
<?php
$number = 81; // global scope
function Scope() {
$number = 5; // local scope
echo "<p>Variable number inside function is: $number</p>";
}
Scope();
echo "<p>Variable number outside function is: $number</p>";
?>
Output:
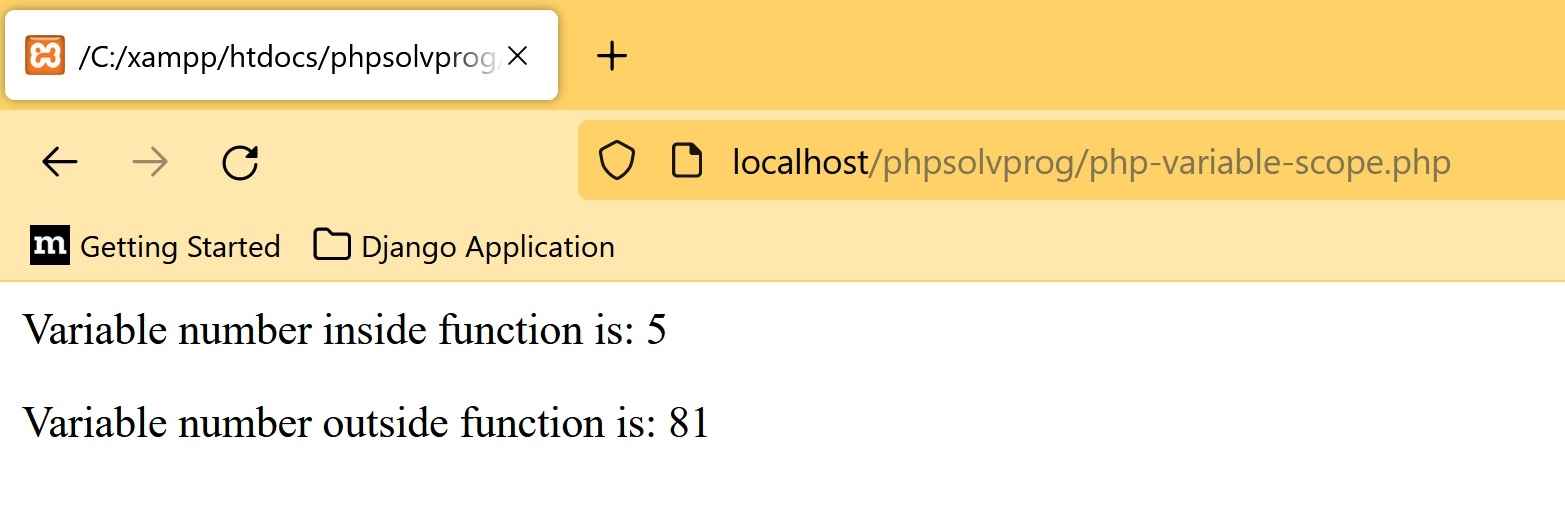
Output: Global variable scope
PHP The global Keyword
To access a global variable from within a function, we need to use the global keyword.
To accomplish this, place the global keyword before the variables (used always inside the function):
Filename: php-variable-scope.php
<?php
$n1 = 81;
$n2 = 9;
function Scope() {
global $n1, $n2;
$n2 = $n1 + $n2;
}
Scope();
echo $n2; // outputs 90
?>
Output:
PHP also keeps track of all global variables in an array known as $GLOBALS[index]. The variable's name and its value is stored in the index. This array is allowed to access from within functions and can be used to update global variables directly.
The example given above can be reused like this producing the same output:
Filename: php-variable-scope.php
<?php
$n1 = 81;
$n2 = 9;
function Scope() {
global $n1, $n2;
$n2 = $n1 + $n2;
}
Scope();
echo $n2; // outputs 90
function Scope2() {
$GLOBALS['n3'] = $GLOBALS['n1'] + $GLOBALS['n2'];
}
Scope2();
echo "<br/>";
echo $n3; // outputs 171
?>
Output:
- 90
- 171
3. PHP The static Keyword / Static variable scope
In PHP features, when a function is completed or executed, then all of its variables are deleted. However, sometimes we want a local variable NOT to be deleted. We may need it for a further task.
To do this, we define a static variable, and use the static keyword. For example:
Filename: php-variable-scope.php
<?php
// static - variable will still have the information it contained from the last time the function was called.
function Scope() {
static $n = 0;
echo $n;
$n++;
}
Scope();
echo "<br/>";
Scope();
echo "<br/>";
Scope();
?>
Output:
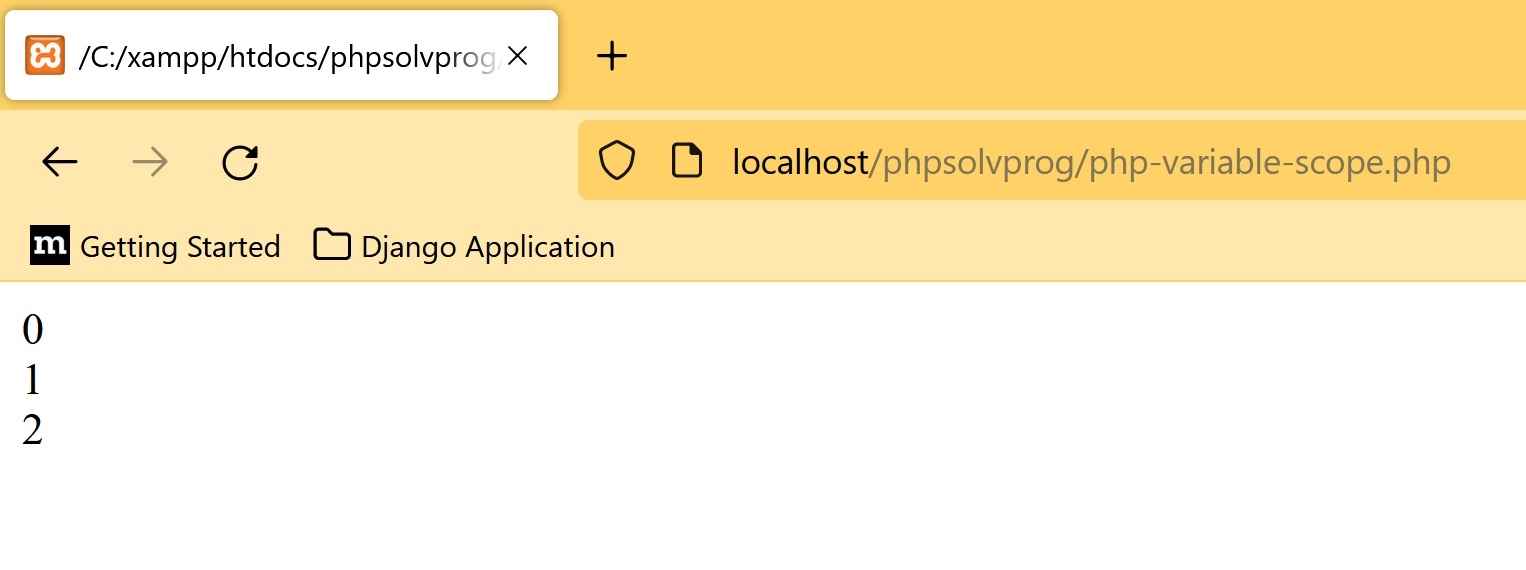
Output: Static variable scope
Then, each time the function is called, that variable will still have the information it contained from the last time the function was called.
Note: The variable is still local to the function.
Conclusion
PHP has three types of variable scopes including local, global, and static variable scope.
Is PHP variable block scope?
When a variable is accessed outside its scope it will cause PHP error Undefined Variable.
Hence, we define the variable scope in a program within which it can be accessed from code.