PHP Data Types
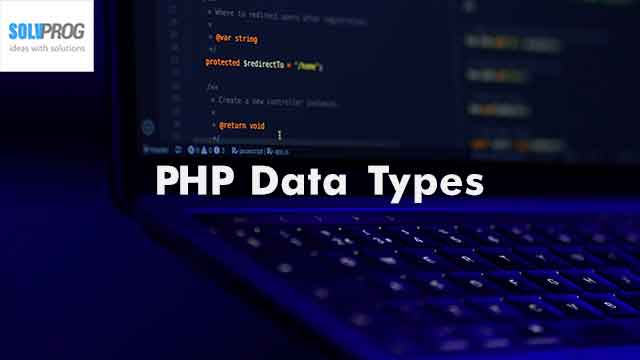
The values assigned to a variable in PHP may be of different data types including string and numeric types to more compound data types like arrays and objects.
Variables can store data of different types, and different data types can do different things.
Data Types in PHP
PHP supports ten primitive types. PHP supports the following data types:
Four scalar types:
- String
- Int
- float (floating-point number, also known as double)
- Boolean
Four compound types:
- Array
- Object
- Callable
- Iterable
And finally two special types:
- Resource
- NULL
1. PHP String
A string is series of characters, where a character is the same as a byte. , like this "Hello world!".
A string can be any text written inside the quotes. You can use single or double quotes:
Example
<?php
$x = "Welcome!";
$y = 'PHP Tutorials on solvprog.com!';
echo $x;
echo "<br>";
echo $y;
?>
2. PHP Integer
An integer data type is a non-decimal number of the set Z = {..., -2, -1, 0, 1, 2, ...}.
Rules for integers:
- An integer must be of at least one digit
- An integer does not have a decimal point
- An integer can be either positive or negative
- Integers can be specified in: decimal (base 10), hexadecimal (base 16), octal (base 8), or binary (base 2) notation.
In the following example $n1 is an integer.
Example
<?php
$n1 = 81;
echo $n1;
?>
3. Float
A float (floating point number) is a number with a decimal point or a number in exponential form.
In the following example $n1 is a float.
Example
<?php
$n1 = 81.365;
echo $n1;
?>
4. PHP Boolean
A boolean expresses a truth value. It can be either true or false.
This is the simplest type with two possible states: (1) TRUE or (0) FALSE.
To specify a bool literal, use the constants true or false. Both are case-insensitive.
Example
<?php
$x1 = true;
$x2 = false;
echo $x1;
echo "<br>";
echo $x2;
?>
5. PHP Array
An array stores multiple values in one single variable.
An array in PHP is actually an ordered map. A map is a type that associates values to keys. This type is optimized for several different uses; it can be treated as an array, list (vector), hash table (an implementation of a map), dictionary, collection, stack, queue, and probably more. As array values can be other arrays, trees and multidimensional arrays are also possible.
An array can be created using the array() language construct. It takes any number of comma-separated key => value pairs as arguments.
Note:
A short array syntax exists which replaces array() with [].
Example
<?php
// Method 1. Using array()
$array1 = array(
"name" => "Sagar",
"age" => 27,
);
// Method 2. Using the short array syntax []
$array2 = [
"name" => "Sagar",
"age" => 27,
];
// Method 3.
$arrayIndex = [ "Sagar", 27 ];
echo $array2[name]; // output: Sagar
echo $array2[age]; // output: 27
echo $arrayIndex[0]; // output: Sagar
echo $arrayIndex[1]; // output: 27
?>
6. PHP Object
Classes and objects are the two main aspects of object-oriented programming.
A class is a template for objects, and an object is an instance of a class.
Example
<?php
class Animal
{
function tiger()
{
echo "Tiger is running..";
}
}
$animal = new Animal;
$animal->tiger();
?>
When the individual objects are created, they inherit all the properties and behaviors from the class, but each object will have different values for the properties.
7. PHP NULL Value
The special null value represents a variable with no value. null is the only possible value of type null.
A variable is considered to be null if:
- 1. it has been assigned the constant null.
- 2. it has not been set to any value yet.
- 3. it has been unset().
There is only one value of type null, and that is the case-insensitive constant null.
Example
<?php
$var = NULL;
?>
Tip: If a variable is created without a value, it automatically assign a value of NULL.
PHP advanced topic - here are 8, 9 and 10 points as follows:
8. PHP Resource
A resource is a special variable, holding a reference to an external resource.
Resources are created and used by special functions.
The special resource type is not an actual data type. It is the storing of a reference to functions and resources external to PHP.
A common example of using the resource data type is a database call.
We will not talk about the resource type here, since it is an advanced topic.
9. Callbacks / Callables
Callbacks can be denoted by the callable type declaration.
Some functions like call_user_func() or usort() accept user-defined callback functions as a parameter. Callback functions can not only be simple functions, but also object methods, including static class methods.
We will cover this in advance topic.
10. Iterables
Iterable can be used as a parameter type to indicate that a function requires a set of values, but does not care about the form of the value set since it will be used with foreach. If a value is not an array or instance of Traversable, a TypeError will be thrown.
We will cover this in advance topic.
The PHP var_dump() function returns the data type and value. Arrays and objects are explored recursively with values indented to show structure.
With var_dump() function we are going to check what its (value) data type is.
Example
<?php
$var1 = 81;
$var2 = "Solvprog.com";
$arr = array(1, 2, array("a", "b", "c"));
var_dump($var1);
echo "<br>";
var_dump($var2);
echo "<br>";
var_dump($arr);
?>
Output:
int(81)
string(12) "Solvprog.com"
array(3) { [0]=> int(1) [1]=> int(2) [2]=> array(3) { [0]=> string(1) "a" [1]=> string(1) "b" [2]=> string(1) "c" } }