PHP Constants
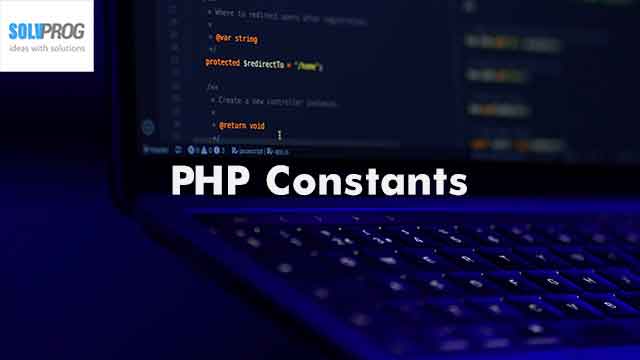
A constant is an identifier (name) like a variable for a simple value. As the name suggests, that value cannot change during the execution of the script (except for magic constants, which aren't actually constants).
Constants in PHP are like variables that contains value, but once they are defined they cannot be changed or undefined.
Constants are case-sensitive.
By convention, constant identifiers are always uppercase.
Note: Prior to PHP 8.0.0, constants defined using the define() function may be case-insensitive.
A valid constant name starts with a letter or underscore (no $ sign before the constant name).
Create a PHP Constant
To create a constant, we use the define() function.
Syntax
define(name, value, case-insensitive)
Parameters:
- name: Specifies the name of the constant
- value: Specifies the value of the constant
- case-insensitive: Specifies whether the constant name should be case-insensitive. by default it takes false.
Example:
Creating a constant with a case-sensitive name:
<?php
define("SOLVPROG", "Welcome to Solvprog.com! PHP Tutorials");
echo SOLVPROG;
?>
Output: a constant with a case-sensitive name
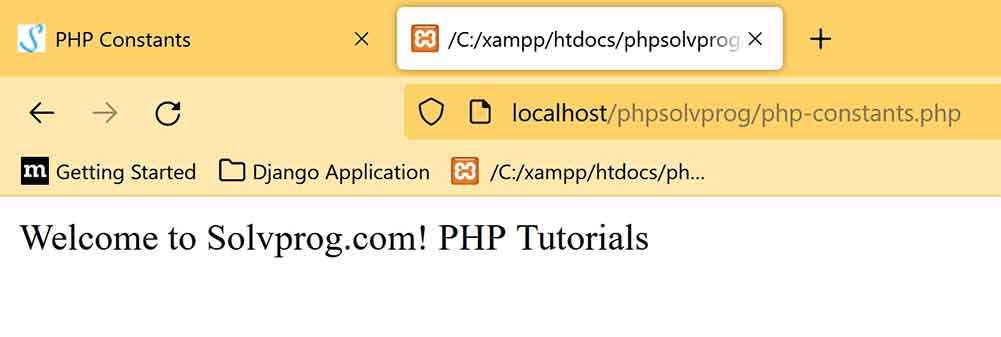
Output: PHP Constants
Example:
Creating a constant with a case-insensitive name:
<?php
define("SOLVPROG", "Welcome to Solvprog.com! PHP Tutorials", true);
echo solvprog;
?>
Output: a constant with a case-insensitive name
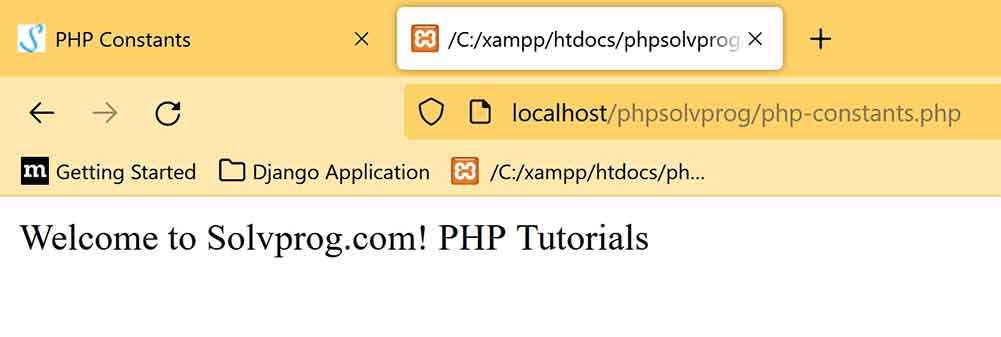
Output: PHP Constants
PHP Constant Arrays
In PHP7, you can create an Array constant using the define() function.
Example:
<?php
define("brands", [
"Google",
"Nike",
"Coca Colla"
]);
echo brands[0]. ", " . brands[1] . " & " . brands[2];
?>
Output:
Google, Nike & Coca Colla
Constants are Global
Constants are automatically global and can be used across the entire script.
Example
This example uses a constant inside a function, and also it is defined outside the function:
<?php
define("SUCCESS", "You define your own success!");
function quotes() {
echo SUCCESS;
}
quotes(); // output: You define your own success!
?>
Output:
You define your own success!