PHP Operators
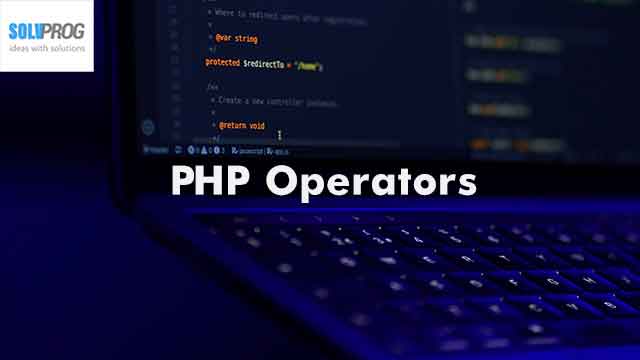
An operator is something that takes one or more values (or expressions) and yields another value (so that the construction itself becomes an expression). Operators are used to perform operations on variables and values.
PHP Operators
PHP define the operators in the following groups:
- 1. Arithmetic Operators
- 2. Assignment Operators
- 3. Comparison Operators
- 4. Incrementing/Decrementing Operators
- 5. Logical operators
- 6. String operators
- 7. Array operators
- 8. Conditional assignment operators
- 9. Operator Precedence
- 10. Bitwise Operators
- 11. Error Control Operators
- 12. Execution Operators
- 13. Type Operators
PHP Arithmetic Operators
The PHP arithmetic operators are used in conjunction with numeric data to execute basic arithmetic operations like as addition, subtraction, multiplication, and so on.
<?php
// $x is a variable that contains value 10
$x = 10;
// $y is a variable that contains value 6
$y = 6;
// PHP Arithmetic Operators
echo "Addition:";
echo $x + $y; // (+) Addition result: Sum of $x and $y
echo "<br/>";
echo "Subtraction:";
echo $x - $y; // (-) Subtraction result: Difference of $x and $y
echo "<br/>";
echo "Multiplication:";
echo $x * $y; // (*) Multiplication result: Product of $x and $y
echo "<br/>";
echo "Division:";
echo $x / $y; // (/) Division result: Quotient of $x and $y
echo "<br/>";
echo "Modulus:";
echo $x % $y; // (%) Modulus result: Remainder of $x and $y
echo "<br/>";
echo "Exponentiation:";
echo $x ** $y; // (**) Exponentiation result: Result of raising $x to the $y'th power
?>
Output:
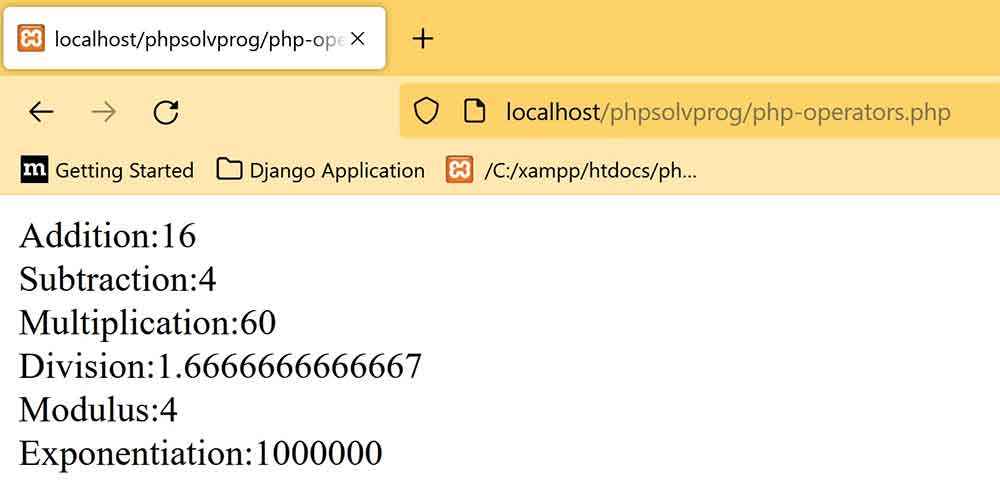
Output: Arithmatic Operators in PHP
PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The most common assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
<?php
// PHP Assignment Operators
echo "Assignment:";
$a = 100;
echo $a; // (=) Assignment result: The left operand gets set to the value of the expression on the right
echo "<br/>";
$x = 10;
$y = 5;
echo "x=".$x.", y=".$y."<br/>";
echo "Addition:";
$x += $y;
echo $x; // (+) Addition $x += $y means $x = $x + $y
echo "<br/>";
echo "Subtraction:";
$x -= $y;
echo $x; // (+) Subtraction $x -= $y means $x = $x - $y
echo "<br/>";
echo "Multiplication:";
$x *= $y;
echo $x; // (*) Multiplication $x *= $y means $x = $x * $y
echo "<br/>";
echo "Division:";
$x /= $y;
echo $x; // (/) Division $x /= $y means $x = $x / $y
echo "<br/>";
echo "Modulus:";
$x %= $y;
echo $x; // (%) Modulus $x %= $y means $x = $x % $y
echo "<br/>";
?>
Output:
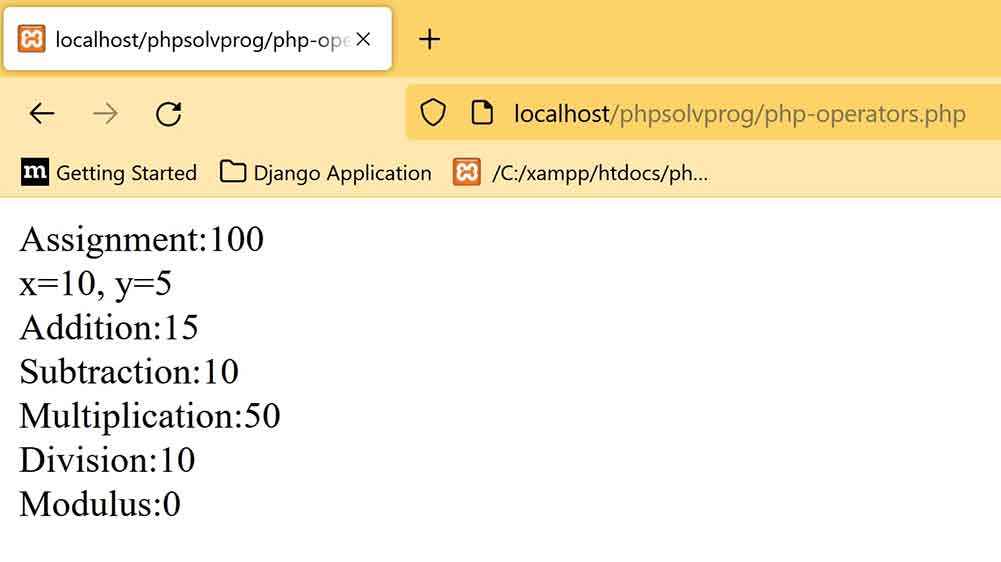
Output: Assignment Operators in PHP
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string).
- == Equal $x == $y Returns the value true if $x is equal to $y
- === Identical $x === $y Returns the value true if $x is equal to $y, and they are of the same type.
- != Not equal $x != $y Returns the value true if $x is not equal to $y
- <> Not equal $x <> $y Returns the value true if $x is not equal to $y
- !== Not identical $x !== $y Returns the value true if $x is not equal to $y, or they are not of the same type
- > Greater than $x > $y Returns the value true if $x is greater than $y
- < Less than $x < $y Returns the value true if $x is less than $y
- >= Greater than or equal to $x >= $y Returns the value true if $x is greater than or equal to $y
- <= Less than or equal to $x <= $y Returns the value true if $x is less than or equal to $y
- <=> Spaceship $x <=> $y Returns an integer less than, equal to, or greater than zero, depending on if $x is less than, equal to, or greater than $y. Introduced in PHP 7.
PHP Increment / Decrement Operators
The PHP increment operators are used to increment / increase a variable's value.
The PHP decrement operators are used to decrement / decrease a variable's value.
- ++$x (Pre-increment) Increments the value $x by one, then returns the value $x
- $x++ (Post-increment) Returns the value $x, then increments the value $x by one
- --$x (Pre-decrement) Decrements the value $x by one, then returns the value $x
- $x-- (Post-decrement) Returns the value $x, then decrements the value $x by one
PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
- And (and) $x and $y Returns the value True if both $x and $y are true
- Or (or) $x or $y Returns the value True if either $x or $y is true
- Xor (xor) $x xor $y Returns the value True if either $x or $y is true, but not both
- And (&&) $x && $y Returns the value True if both $x and $y are true
- Or (||) $x || $y Returns the value True if either $x or $y is true
- Not (!) !$x Returns the value True if $x is not true
PHP String Operators
PHP has two operators that are specifically built for strings to use.
- . Concatenation $txt1 . $txt2 It means Concatenation of $txt1 and $txt2
- .= Concatenation assignment $txt1 .= $txt2 Appends (addition) the variables value of $txt2 to $txt1
PHP Array Operators
The PHP array operators are used to compare arrays.
- + Union $x + $y Union of $x and $y
- == Equality $x == $y Returns true if $x and $y have the same key/value pairs
- === Identity $x === $y Returns true if $x and $y have the same key/value pairs in the same order and of the same types
- != Inequality $x != $y Returns true if $x is not equal to $y
- <> Inequality $x <> $y Returns true if $x is not equal to $y
- !== Non-identity $x !== $y Returns true if $x is not identical to $y
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value based on the conditions:
- ?: Ternary $x = expr1 ? expr2 : expr3 Returns the value of $x. The value of $x is expr2 if expr1 = TRUE. The value of $x is expr3 if expr1 = FALSE
- ?? Null coalescing $x = expr1 ?? expr2 Returns the value of $x. The value of $x is expr1 if expr1 exists, and is not NULL. If expr1 does not exist, or is NULL, the value of $x is expr2. Introduced in PHP 7