PHP If, else and elseif Conditional Statements
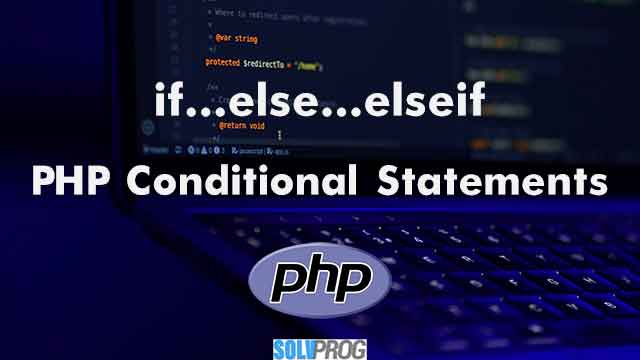
Conditional statements are used to perform different actions based on different conditions. In PHP we have the following conditional statements that we are going to cover in this tutorial - if statement, if...else statement and if...elseif...else statement.
PHP Conditional Statements
When writing code, you frequently want to do different actions based on for different conditions. To do this, you can use conditional statements in your code.
In PHP we have the following conditional statements:
- if statement - executes a block of code if one condition is true
- if...else statement - executes a block of code if a condition is true and another block of code with else executes if the condition is false for the if statements.
- if...elseif...else statement - executes different codes for more than two conditions
- switch statement - selects one of many blocks of code to be executed
if Statement in PHP
The if statement executes some code only if the given condition / expressions is true.
PHP features an if structure that is similar to that of C:
Syntax
if (condition) {
code to be executed if condition is true;
}
Condition is evaluated to its Boolean value. If the condition evaluates to true, then PHP will execute statement, and if it evaluates to false - therefore, it'll ignore it.
Example #1
Output the following statement "The time is less than 21 hour today!" - if the current time (HOUR) is less than 21 (we will be using date("H") ):
<?php
$time = date("H");
if ($time < "21") {
echo "The time is less than 21 hour today!";
}
?>
Example #2
Using variables to compare their values if the condition one variable is greater than another variable. Output the following "a is bigger than b"
<?php
$a = 10;
$b = 9;
if ($a > $b)
echo "a is bigger than b";
?>
Example #3
Easy way to execute conditional html / javascript / css / other language code with php if statement:
<?php if (condition){ ?>
html code to run if condition is true
<?php } ?>
Example #4 Method 1.
Nested if statement
You can have 'nested' if statements withing a single if statement, using additional parenthesis.
<?php
if( $a == 1 || $a == 2 ) {
if( $b == 3 || $b == 4 ) {
if( $c == 5 || $ d == 6 ) {
//Do something here.
}
}
}
?>
Example #4 Method 2.
OR, you could just simply do this:
<?php
if( ($a==1 || $a==2) && ($b==3 || $b==4) && ($c==5 || $c==6) ) {
//do that something here.
}
?>
if...else Statement in PHP
The if...else statement allows you to execute one block of code if the specified condition is evaluates to true and another block of code if it evaluates to false. It's Syntax can be given, like this:
Syntax
if (condition) {
code to be executed if condition is true;
} else {
code to be executed if condition is false;
}
Example #1
Output "It's a good day!" if the current time is less than 21, and "It's a good night!" otherwise (using date("H") ):
<?php
$time = date("H");
if ($time < "21") {
echo "It's a good day!";
} else {
echo "It's a good night!";
}
?>
Example #2
Easy way to execute conditional html / javascript / css / other language code with php if else:
<?php if (condition): ?>
html code to run if condition is true
<?php else: ?>
html code to run if condition is false
<?php endif ?>
if...elseif...else Statement in PHP
The if...elseif...else statement executes different specific codes for more than two conditions. It is used for combination of multiple if...else statements.
Syntax
if (condition) {
code to be executed if this condition is true;
} elseif (condition) {
code to be executed if first condition is false and this condition is true;
} else {
code to be executed if all conditions are false;
}
Example #1
The following example will output "It's a nice weekend! enjoy" if the current day is Friday, and "It's a nice Sunday! enjoy" if the current day is Sunday, otherwise it will output "It's a nice day! busy"
<?php
$day = date("D");
if($day == "Fri"){
echo "It's a nice weekend! enjoy";
} elseif($day == "Sun"){
echo "It's a nice Sunday! enjoy";
} else{
echo "It's a nice day! busy";
}
?>
<?php
$a = 10;
$b = 10;
/* Incorrect Method: */
if ($a > $b):
echo $a." is greater than ".$b;
else if ($a == $b): // Will not compile.
echo "The above line causes a parse error.";
endif;
/* Correct Method: */
if ($a > $b):
echo $a." is greater than ".$b;
elseif ($a == $b): // Note the combination of the words.
echo $a." equals ".$b;
else:
echo $a." is neither greater than or equal to ".$b;
endif;
?>