PHP switch Statement
Updated: April 19th, 2022, 10:37:38 IST
Published:
April 19th, 2022
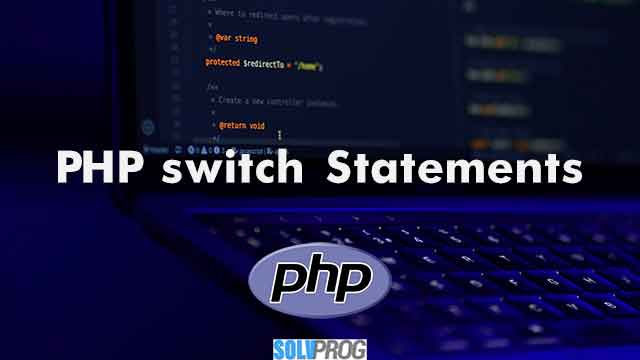
A switch statement is similar to a series of IF statements on the same expression. In many cases, you may wish to compare the same variable (or expression) to a given set of values and execute a different piece of code based on which value it equals. This is exactly the objective of the switch statement.
switch Statement
Syntax
switch (n) {
case label1:
code to be executed if n=label1;
break;
case label2:
code to be executed if n=label2;
break;
case label3:
code to be executed if n=label3;
break;
...
default:
code to be executed if n is different from all labels;
}
Here's how it works:
- First, there is a single expression n (usually a variable) that is evaluated once.
- The value of the expression is then compared to the values in the structure for each case.
- If a match is found, the code associated with that case is performed.
- Use break to prevent the code from automatically moving on to the next scenario.
- If no match is discovered, the default statement is used.
Example #1
It's use is similar to elseif statement we can try both ways to execute the same output.
<?php
if ($i == 0) {
echo "i equals 0";
} elseif ($i == 1) {
echo "i equals 1";
} elseif ($i == 2) {
echo "i equals 2";
}
switch ($i) {
case 0:
echo "i equals 0";
break;
case 1:
echo "i equals 1";
break;
case 2:
echo "i equals 2";
break;
}
?>
Example #2
<?php
$favday = "friday";
switch ($favday) {
case "monday":
echo "My favorite day is monday!";
break;
case "tuesday":
echo "My favorite day is tuesday!";
break;
case "friday":
echo "My favorite day is friday!";
break;
default:
echo "My favorite day is neither monday, tuesday, nor friday!";
}
?>
It should be noted that, unlike in some other languages, the continue statement applies to switch and functions similarly to the break statement. continue is used if you have a switch within a loop and want to continue to the next iteration of the outer loop.
Some important note on switch Statement:
- To avoid errors, it is critical to understand how the switch statement is performed.
- The switch statement is executed one line at a time (actually, statement by statement).
- No code is performed at the beginning.
- PHP begins executing the statements only when a case statement is found whose expression evaluates to a value that matches the value of the switch expression.
- PHP executes the statements until the end of the switch block or the first time it encounters a break statement.
- If you do not include a break statement at the end of a case's statement list, PHP will continue to execute the statements in the next case.