JavaScript Variables (var, let, const) - Javascript Tutorial
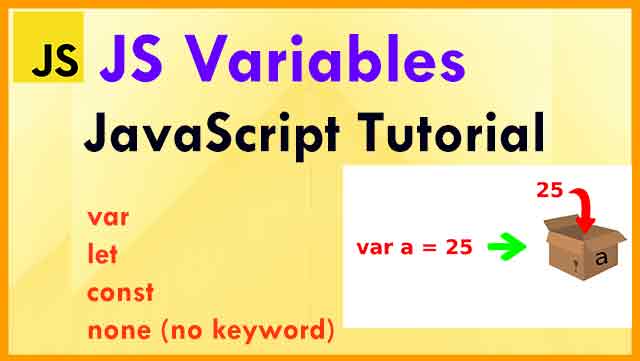
A JavaScript variable is basically a name of storage location and the data or information is stored in it. There are two ways in which a variable can be used in JavaScript: local variable and global variable.
4 Ways to Declare a JavaScript Variable:
- Using var
- Using let
- Using const
- Using nothing
What are Variables in JavaScript?
Variables are containers for storing data (or any kind of info.) storing data values.
Let's take an example of, a, b, and z, are variables, declared with the var keyword
<!DOCTYPE html>
<html>
<body>
<h1>JavaScript Variables</h1>
<p>In this example, a, b, and z are variables.</p>
<p id="number-id"></p>
<script>
var a = 81;
var b = 61;
var z = a + b;
document.getElementById("number-id").innerHTML =
"The value of z is: " + z;
</script>
</body>
</html>
In this example, a, b, and z, are variables, declared with the let keyword:
<!DOCTYPE html>
<html>
<body>
<h1>JavaScript Variables</h1>
<p>In this example, a, b, and z are variables.</p>
<p id="number-id"></p>
<script>
let a = 81;
let b = 61;
let z = a + b;
document.getElementById("number-id").innerHTML =
"The value of z is: " + z;
</script>
</body>
</html>
In this example, a, b, and z, are undeclared variables (we did not declare any keyword just simply giving them name to store a value):
<!DOCTYPE html>
<html>
<body>
<h1>JavaScript Variables</h1>
<p>In this example, a, b, and z are variables.</p>
<p id="number-id"></p>
<script>
a = 81;
b = 61;
z = a + b;
document.getElementById("number-id").innerHTML =
"The value of z is: " + z;
</script>
</body>
</html>
In the above given examples you can simply understand how a variable is being used and the results are same for all types of variables.
- a stores the value 81
- b stores the value 61
- z stores the value 142
When to Use JavaScript var?
Always declare JavaScript variables with var,let, or const.
* The var keyword is used in all JavaScript code from 1995 to 2015.
* The let and const keywords were added to JavaScript in 2015.
If you want your code to run in older browser, you must use var. otherwise, it's recommended to use let and const for declaring a variable.
When to Use JavaScript const?
If you want to use variable according to basic rule: always declare variables with const.
If you think the value can change for the variable declared, we use let keyword.
In this example, item1, item2, and total, are variables:
const item1 = 5;
const item2 = 6;
let total = item1 + item2;
Note: Variables are containers for storing values.
JavaScript Identifiers
All JavaScript variables must be identified with unique names.
These unique names are called identifiers.
The basic rules for putting up names for variables (unique identifiers) are:
- Names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter
- Names can also begin with $ (dollar sign) and _ (underscore)
- Names are case sensitive (a and A are different variables)
- Reserved words (like JavaScript keywords - var, let) cannot be used as names.
JavaScript Data Types
JavaScript variables can hold numbers like 100 and text values like "John Doe". Most of the time we use numbers and text values (strings) as data types for storing value.
JavaScript can handle many types of data, but for now, just use numbers and strings.
Strings are written inside double or single quotes. Numbers are written without quotes.
If you put a number in quotes, it will be treated as a text string.
const pi = 3.14;
let person_name = "Sagar Kalyan";
let age = 26;
Make a note: On Variables in JS
- Declaring a variable
- Assigning value to a variable
- One Statement, Many Variables
- Re-Declaring JavaScript Variables:
- var - we can re-declare (it will have the same value, as previously assigned.)
- let - we cannot re-declare
- JavaScript Arithmetic (addition, subtraction)
- Javascript concatination (+)