JavaScript Let Keyword
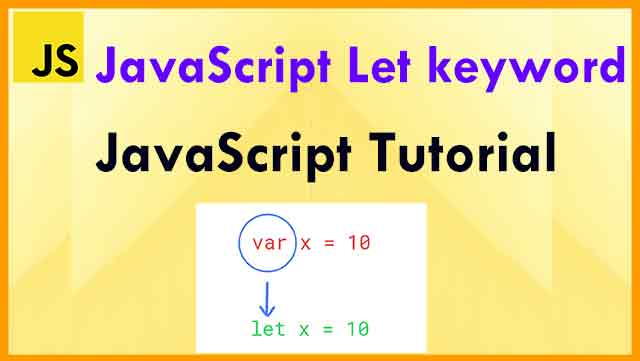
Let is a keyword in JavaScript that is used to declare a block scoped variable. In JavaScript, the var keyword is often used to create a variable that is handled as a typical variable, however variables declared using the let keyword are block scoped.
In comparison to the var keyword, which declares a variable globally or locally to an entire function regardless of block scope, let allows you to define variables that are limited to the scope of the block statement or expression on which it is used.
Key Points:
- The let keyword first appeared in ES6 (2015).
- Let-defined variables cannot be redeclared.
- Variables defined using let must be declared before they could be used.
- Variables using the keyword let have Block Scope.
1. Syntax of let
The following is the syntax for declaring a variable with the let keyword:
let variable_name;
2. Let keyword in JavaScript cannot be Re-declared
Let-defined variables cannot be redeclared.
With let you can not do this:
let n = "Sagar InspireMe";
let n = 10;
// SyntaxError: 'n' has already been declared
3. Must Be Declared Before Using let keyword.
Using a let variable before declaring it, results in a ReferenceError:
myName = "InspireMe";
let myName = "Sagar";
// It's NOT allowed to use like this..
The correct way to use let keyword is:
// 1. First Method
let myName = "Sagar InspireMe";
// 2. Second Method
let myName;
myName = "Sagar InspireMe";
4. Let keyword have Block Scope
- JavaScript had only Global Scope and Function Scope prior to ES6 (2015).
- Let and const are two crucial new JavaScript keywords introduced in ES6.
- In JavaScript, these two keywords let and const provide Block Scope.
- Variables declared within a block are not accessible from outside the block.
Example with code:
{
let n = 2;
// n declared with let keyword inside a block is used.
}
// n can NOT be used here
Browser Support The let keyword is not fully supported in Internet Explorer 11 or earlier.
Redeclaring a variable within the same block is not permitted when using let.
var n = 2; // Allowed
let n = 3; // Not allowed
{
let n = 2; // Allowed
let n = 3 // Not allowed
}
{
let n = 2; // Allowed
var n = 3 // Not allowed
}
Redeclaring a variable with let, in another block
let n = 2; // Allowed
{
let n = 3; // Allowed
}
{
let n = 4; // Allowed
}
5. Let Hoisting
Variables defined with var are hoisted to the top of the list and can be initialized at any time.
In other words, you can use the variable before it is defined.
// It is Ok to use..
myName = "Volvo";
var myName;
Variables defined using let are hoisted to the top of the block as well, but they are not initialized.
Using a let variable before it results in a ReferenceError:
carName = "Saab";
let carName = "Volvo";
// It's NOT allowed to use like this..