JavaScript Const Keyword
Updated: May 31st, 2022, 03:56:31 IST
Published:
May 31st, 2022
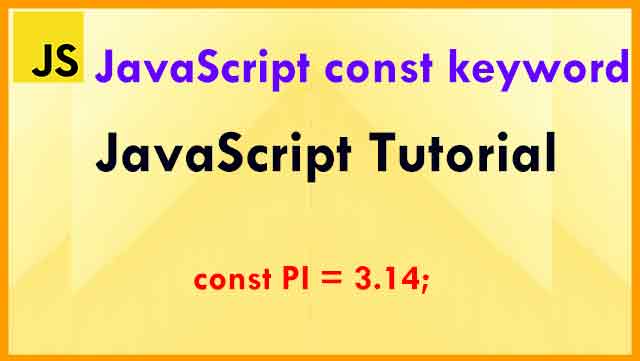
The const keyword was added in ES6 and is used to define a new variable in JavaScript. The var keyword was often used to declare a JavaScript variable, earlier phase before ES6. When you declare a variable and don't want to modify its value for the duration of the program, you can use the const keyword.
- The const keyword was added in ES6 (2015).
- Variables using the const keyword cannot be redeclared.
- Const-defined variables cannot be reassigned.
- Variables defined with const have Block Scope.
A const variable cannot be reassigned.
const PI = 3.141592653589793;
PI = 3.14; // This will give an error
PI = PI + 10; // This will also give an error
When declaring const variables in JavaScript, they must be given a value (must be assigned).
// Declaring and Assigning a value together
const PI = 3.14159265359;
OR
// 1. Declaring
const PI;
// 2. Assigning a value
PI = 3.14159265359;
When should you use JavaScript const?
As a general guideline, unless you know the value will change, always declare a variable using const.
When declaring: use const.
- A new Array
- A new Object
- A new Function
- A new RegExp
Example: Constant Arrays
You can change the elements of a constant array:
// You can create a constant array:
const cars = ["Audi", "Bentley", "BMW"];
// You can change an element:
cars[0] = "Toyota";
// You can add an element:
cars.push("Maruti Swift");
But you can NOT reassign the array:
const cars = ["Audi", "Bentley", "BMW"];
cars = ["Toyota", "Volvo", "Audi"]; // ERROR will be generated.
Block Scope for const keyword
Declaring a variable with const is similar to let when it comes to Block Scope.
The n declared in the block, in this example, is not the same as the n declared outside the block.
const n = 10;
// Here n is 10
{
const n = 2;
// Here n is 2
}
// Here n is 10
Const Hoisting
Variables defined with const are also hoisted to the top, but not initialized.
Meaning: Using a const variable before results in a ReferenceError:
// const Not allowed to use like this
alert (myName);
const myName = "Sagar InspireMe";
// Correct Method to do is:
// First declare then assign value and then use it as a block scope.
const myName = "Sagar InspireMe";
alert (myName);