JavaScript Operators - JavaScript Tutorial
Updated: June 17th, 2022, 02:22:37 IST
Published:
June 17th, 2022
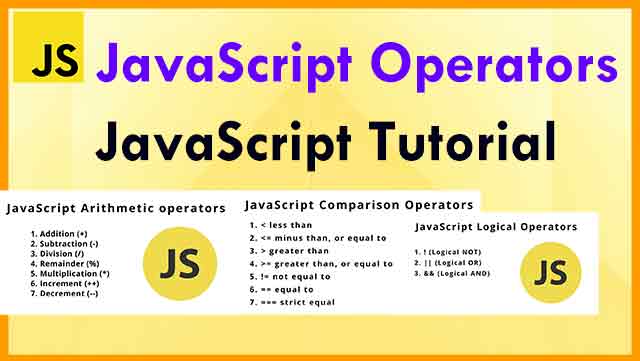
An operator is a program that performs an operation on a single or more operands (data values) and returns a result. In 1 + 2, for example, the + sign is an operator, and 1 is the left side operand and 2 is the right side operand. JavaScript includes operators similar to other languages.
In this tutorial, you will learn about different operators available in JavaScript and how to use them with the help of examples.
- 1. JavaScript Arithmetic Operators
- 2. JavaScript Assignment Operators
- 3. JavaScript Comparison Operators
- 4. JavaScript Logical Operators
- 5. JavaScript Type Operators
- 6. JavaScript Bitwise Operators
- - JavaScript String Operators
- - Adding Strings and Numbers
What is an Operator?
In JavaScript, an operator is a special symbol used to perform operations on operands (values and variables). For example,
2 + 3;
// Output is: 5
Here + is an operator that performs addition, and 2 and 3 are operands.
// 1: Arithmetic operators in JavaScript
let x = 7;
let y = 3;
// addition
console.log('x + y = ', x + y); // 10
// subtraction
console.log('x - y = ', x - y); // 4
// multiplication
console.log('x * y = ', x * y); // 21
// division
console.log('x / y = ', x / y); // 2.3333333333333335
// remainder
console.log('x % y = ', x % y); // 1
// increment
console.log('++x = ', ++x); // x is now 8
console.log('x++ = ', x++); // prints 8 and then increased to 9
console.log('x = ', x); // 9
/*
At this moment,
x is equal to 9
y is equal to 3
*/
// decrement
console.log('--x = ', --x); // x is now 8
console.log('x-- = ', x--); // prints 8 and then decreased to 7
console.log('x = ', x); // 7
/*
At this moment,
x is equal to 7
y is equal to 3
*/
//exponentiation
console.log('x ** y =', x ** y); // 343
// 2: Assignment Operators in JavaScript
let a = 2;
let b = 6;
/*
a = b
a = a + b (+=)
a = a - b (-=)
a = a * b (*=)
a = a / b (/=)
a = a % b (%=)
a = a ** b (**=)
*/
// 3: Comparison operators in JavaScript
// equal to operator
console.log(2 == 2); // true
console.log(2 == '2'); // true
// not equal to operator
console.log(3 != 2); // true
console.log('hello' != 'Hello'); // true
// strict equal to operator
console.log(2 === 2); // true
console.log(2 === '2'); // false
// strict not equal to operator
console.log(2 !== '2'); // true
console.log(2 !== 2); // false
/*
less than
<
greater than
>
>= greater than or equal to
<= less than or equal to
? ternary operator
*/
// 4: Logical operators in JavaScript
// logical AND
console.log(true && true); // true
console.log(true && false); // false
// logical OR
console.log(true || false); // true
// logical NOT
console.log(!true); // false
// 5: Type operators in JavaScript
/*
typeof - Returns the type of a variable
instanceof - Returns true if an object is an instance of an object type
*/
// 6: Bitwise operators in JavaScript
/*
& - Bitwise AND (x & y)
| - Bitwise OR (x | y)
^ - Bitwise XOR (x ^ y)
~ - Bitwise NOT (~x)
<< - Left shift (x << y)
>> - Sign-propagating right shift (x >> y)
>>> - Zero-fill right shift (x >>> y)
*/
// 7: String operators in JavaScript
let firstName = "Sagar";
let lastName = "InspireMe";
let year = 2022;
let text3 = firstName + " " + lastName;
let text4 = firstName + " " + lastName + " - " + year;