JavaScript Data Types - JavaScript Tutorial
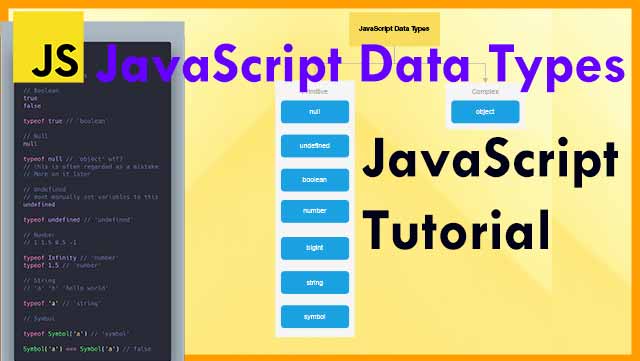
JavaScript variables can hold different data types: numbers, strings, objects and more. In this tutorial, you will learn about the various data types available in JavaScript with the help of examples.
There are different types of data that we can use in a JavaScript program. For example,
const x = 9;
const y = "Hello";
Here,
9 is an integer data type.
"Hello" is a string data type.
What are the data types in JavaScript?
In this tutorial, I will explain with examples 8 data types in JavaScript.
- Number type.
- String type.
- Boolean type.
- Null type.
- Undefined type.
- BigInt type.
- Symbol type.
- Object type.
Here, all data types except Object are primitive data types, whereas Object is non-primitive.
Note: The Object data type (non-primitive type) can store collections of data, whereas primitive data type can only store a single data.
A value in JavaScript is always of a certain type. For example, a string or a number.
JavaScript Types are Dynamic
JavaScript has dynamic types. This means that the same variable can be used to hold different data types:
Example:
let n; // Now n is undefined
n = 9; // Now n is a Number
n = "Sagar"; // Now n is a String
1. Number
// Number Data Type
let n1 = 34.00; // number with decimals
let n2 = 34; // number without decimals
2. String
let str = "Hello"; // string with double quotes
let str2 = 'Single quotes are ok to use also'; // string with single quotes
let phrase = `can embed another ${str}`; // string with backticks
In JavaScript, there are 3 types of quotes.
- Double quotes: "Hello".
- Single quotes: 'Hello'.
- Backticks: `Hello`.
Example
let name = "Sagar";
// embed a variable
alert( `Hello, ${name}!` ); // Hello, Sagar!
// embed an expression
alert( `the result is ${1 + 2}` ); // the result is 3
/* testing the expression inside double quotes using ${…}
is not evaluated here.
*/
alert( "the result is ${1 + 2}" ); // the result is ${1 + 2} (double quotes do nothing)
3. BigInt
// the "n" at the end means it's a BigInt
const bigInt = 1234567890123456789012345678901234567890n;
4. Boolean (logical type)
The boolean type has only two values: true and false.
let x = 2;
let y = 2;
let z = 6;
(x == y) // Returns true
(x == z) // Returns false
// Boolean values also come as a result of comparisons:
let isGreater = 4 > 1;
console.log( isGreater );
// true (the comparison result is: "yes")
5. The "null" value
The special null value does not belong to any of the types described above.
let numb = null;
It’s a special value which represents "nothing", "empty" or "value unknown".
The code above states that numb is unknown.
6. The "undefined" value
The meaning of undefined is "value is not assigned".
let n;
alert(n); // shows "undefined"
7. & 8. Objects and Symbols
The Object data type (non-primitive type) can store collections of data, whereas primitive data type can only store a single data.
// objects
const person = {firstName:"Sagar", lastName:"Kalyan", age:81, eyeColor:"black"};
The symbol type is used to create unique identifiers for objects.
8. The typeof Operator
typeof undefined // "undefined"
typeof 0 // "number"
typeof 10n // "bigint"
typeof true // "boolean"
typeof "Sagar" // "string"
typeof Symbol("id") // "symbol"
typeof Math // "object" (1)
typeof null // "object" (2)
typeof alert // "function" (3)
In Conclusion: Data Types in JavaScript
There are 8 basic data types in JavaScript.
number for numbers of any kind: integer or floating-point, integers are limited by ±(253-1).
bigint is for integer numbers of arbitrary length.
string for strings. A string may have zero or more characters, there’s no separate single-character type.
boolean for true/false.
null for unknown values - a standalone type that has a single value null.
undefined for unassigned values - a standalone type that has a single value undefined.
object for more complex data structures.
symbol for unique identifiers.