PHP Arrays
Updated: September 29th, 2022, 01:12:18 IST
Published:
September 28th, 2022
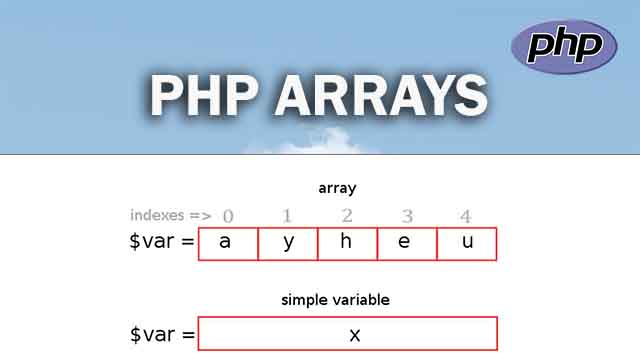
An array is a special variable, which can hold more than one value at a time. An array can hold many values under a single name, and you can access the values by referring to an index number. We will learn to create an array in PHP.
Arrays in PHP
An array stores multiple values in one single variable:
<?php
$names = array("Hailey", "Ana", "Sharlene");
echo "I like " . $names[0] . ", " . $names[1] . " and " . $names[2] . ".";
?>
Output will be something like this:
I like Hailey, Ana and Sharlene.
What is an Array?
An array is a collection of items of same data type stored at contiguous memory locations.
In simple terms, an array is a special variable, which can hold more than one value at a time.
An array can hold many values under a single name, and you can access the values by referring to an index number.
Create an Array in PHP
In PHP, the array() function is used to create an array:
array();
What are the 3 types of arrays in PHP?
In PHP, there are three types of arrays:
- Indexed arrays - Arrays with a numeric index
- Associative arrays - Arrays with named keys
- Multidimensional arrays - Arrays containing one or more arrays
Applications on Array
- Array stores data elements of the same data type.
- Arrays are used when the size of the data set is known.
- Used in solving matrix problems.
- Applied as a lookup table in computer.
- Databases records are also implemented by the array.
- Helps in implementing sorting algorithm.
- The different variables of the same type can be saved under one name.
- Arrays can be used for CPU scheduling.
- Used to Implement other data structures like Stacks, Queues, Heaps, Hash tables, etc.
How to get the length of an array in PHP?
The count() function is used to return the length (the number of elements) of an array.
Example:
<?php
$numbers = array("One", "Two", "Three");
echo count($numbers);
?>
Output:
3