PHP Associative Arrays - PHP Arrays
Updated: September 29th, 2022, 01:07:10 IST
Published:
September 28th, 2022
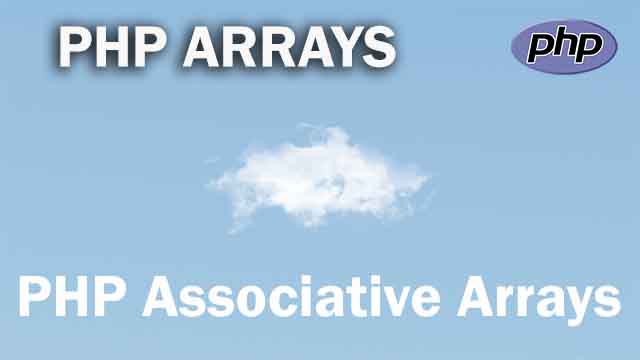
Associative arrays are arrays that use named keys that you assign to them. Key-value pairs are stored in associative arrays. A numerically indexed array might not be the ideal option.
For instance, to record a student's marks from several subjects in an array. Instead, we could utilize the names of the relevant subjects as the keys in our associative array and their respective marks earned as the value.
Example:
<?php
/* First method to create an associate array. */
$student_one = array("Maths"=>85, "Physics"=>70,
"Chemistry"=>66, "English"=>95,
"Computer"=>99);
/* Second method to create an associate array. */
$student_two["Maths"] = 85;
$student_two["Physics"] = 70;
$student_two["Chemistry"] = 66;
$student_two["English"] = 95;
$student_two["Computer"] = 99;
/* Accessing the elements directly */
echo "Marks for student one is:<br/>";
echo "Maths:" . $student_two["Maths"], "<br/>";
echo "Physics:" . $student_two["Physics"], "<br/>";
echo "Chemistry:" . $student_two["Chemistry"], "<br/>";
echo "English:" . $student_one["English"], "<br/>";
echo "Computer:" . $student_one["Computer"], "<br/>";
?>
Output:
Marks for student one is:
Maths:85
Physics:70
Chemistry:66
English:95
Computer:99
There are two ways to create an associative array:
$age = array("John"=>"26", "Sam"=>"17", "Jazz"=>"23");
OR
$age['John'] = "26";
$age['Sam'] = "17";
$age['Jazz'] = "23";
The named keys can then be used in a script as:
<?php
$age = array("John"=>"26", "Sam"=>"17", "Jazz"=>"23");
echo "John is " . $age['John'] . " years old.";
?>
Output:
John is 26 years old.
Loop Through an Associative Array
To loop through and print all the values of an associative array, you could use a foreach loop, like this:
Example:
<!DOCTYPE html>
<html>
<body>
<?php
$age = array("John"=>"26", "Sam"=>"17", "Jazz"=>"23");
foreach($age as $key => $key_value) {
echo "Key=" . $key . ", Value=" . $key_value;
echo "<br>";
}
?>
</body>
</html>
Output:
Key=John, Value=26
Key=Sam, Value=17
Key=Jazz, Value=23
Creating an associative array of mixed types
Example
<?php
/* Creating an associative array of mixed types */
$arr["xyz"] = 5;
$arr[101] = "abcd";
$arr[21.25] = 1000;
$arr["abc"] = "pqrstuvwxyz";
/* Looping through an array using foreach */
foreach ($arr as $key => $val){
echo $key."==>".$val."<br/>";
}
?>
Output:
xyz==>5
101==>abcd
21==>1000
abc==>pqrstuvwxyz