Python def Keyword
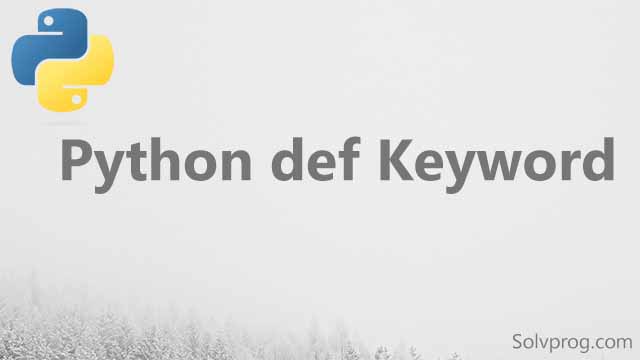
In Python, "def" is a keyword used to define a function. It's followed by the name of the function and a set of parentheses containing any parameters that the function will take. The function body, which is indented under the "def" statement, contains the code that will be executed when the function is called. The return statement is used to specify what value the function should return. When the function is called with an argument, the code inside the function body is executed and the function returns the specified value.
Def Keyword in Python
Syntax:
def function_name:
function definition statements...
When you write a function in Python, you use the keyword "def" followed by the name of the function and any parameters in parentheses. You then use a colon to indicate the start of the function body, which needs to be indented. The code inside the function body determines what value the function will return when it is called with an argument. Once you've defined the function, you can call it with an argument to get the desired output.
Here's a simple example of a function defined using the "def" keyword in Python:
Example
def greet(name):
"""
This function takes a name as a parameter and prints a greeting.
"""
print("Hello, " + name + "!")
# Call the function
greet("Alice")
In this example, we define a function called greet that takes one parameter, name. The function prints a greeting using the print() statement, which includes the value of the name parameter. Finally, we call the greet() function with the argument "Alice", which produces the output "Hello, Alice!" on the screen.
Example
# Define a function to subtract two numbers
def subtract(x, y):
difference = x - y
return difference
# Set the values of a and b
a = 90
b = 50
# Call the subtract function with a and b as arguments
result = subtract(a, b)
# Print the result of the subtraction
print("The difference between", a, "and", b, "is", result)
Output:
The value of a is: 90
The value of b is: 50
The difference between 90 and 50 is 40
Key points about the def function keyword in Python:
- def is a keyword used to define a function in Python.
- The syntax for defining a function is def function_name(parameters):.
- The function name should be descriptive and meaningful, and follow the same naming conventions as variables (e.g. using lowercase letters and underscores to separate words).
- The parameters of a function are values that are passed to the function when it's called. They're placed inside the parentheses after the function name.
- The body of the function is indented under the def statement, and contains the code that will be executed when the function is called.
- The return statement is used to specify what value the function should return. If a function doesn't have a return statement, it will return None.
- Functions can be called with arguments, which are the values that are passed to the function as parameters when it's called.
- Functions can be defined and called from within other functions or from the main program code.
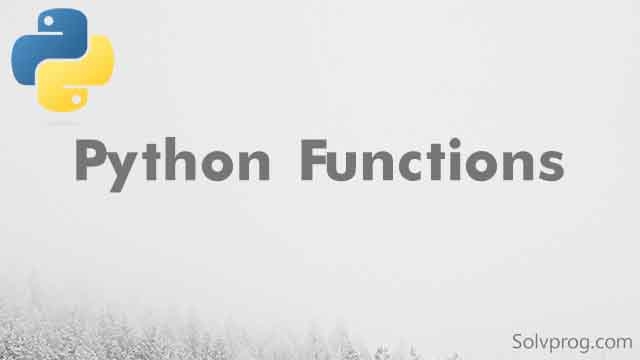
Functions are an essential aspect of any programming language. In Python, a function is a block of reusable code that performs a specific task. A... read more